In this OpenCV Python tutorial, we explain
- How to read images from PNG or JPG files in Python and OpenCV.
- How to display images in OpenCV and Python.
- How to resize images in OpenCV and Python.
- How to save images to files in OpenCV and Python
This website tutorial is based on the image given below.
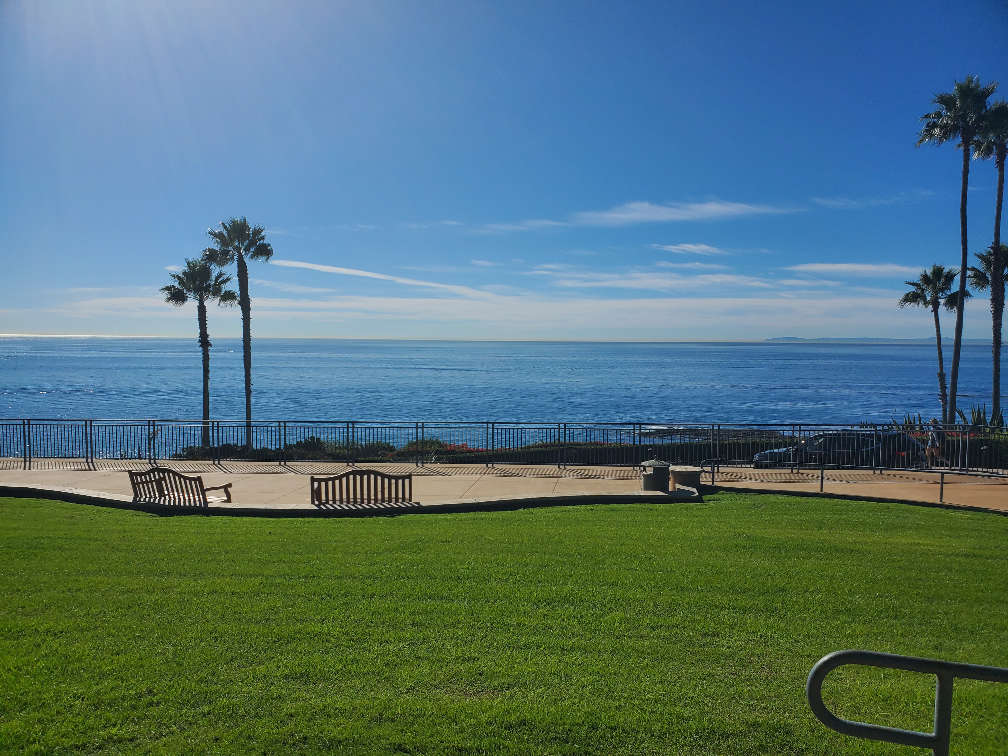
The reader of this website tutorial can freely download this image (you have Dr. Haber’s permission to download the image), or he/she can use any other image in the JPG or PNG file format.
The YouTube tutorial accompanying this webpage tutorial is given below.
How to read images from PNG or JPG files in Python and OpenCV.
The Python script given below reads an image from the file “photo1.jpg” and investigates the basic image properties in OpenCV.
# import OpenCV
import cv2
# Load the image from the file
imageOriginal = cv2.imread('photo1.jpg')
# for the PNG file format use the same function
# only with a different file
# name and PNG extension
# let see the shape of the image
rowOriginal,colOriginal,cOrignal=imageOriginal.shape
# It should be kept in mind that
# OpenCV loads images in the BGR format
# B - blue, G - green, R- red
# and not in the RGB format!
# we can get the channels by typing
# blue channel
imageOriginal[:,:,0]
# green channel
imageOriginal[:,:,1]
# red channel
imageOriginal[:,:,2]
# we can check the max and min values
imageOriginal[:,:,0].max()
imageOriginal[:,:,0].min()
# let us check the data type
imageOriginal[:,:,0].dtype
We import the complete OpenCV library by typing
import cv2
Note that the same import command is valid for all versions of OpenCV (for version 2,3,4, and 5). We read the image from the file by using
imageOriginal = cv2.imread('photo1.jpg')
The input of the function “cv2.imread(‘photo1.jpg’)” is the name of the image file with the extension. You can use the same function to open other types of image files, such as PNG files for example. The image is stored in the NumPy array called imageOriginal.
We can investigate the shape of the loaded image by typing
rowOriginal,colOriginal,cOrignal=imageOriginal.shape
The returned shape is (3024, 4032, 3). Let us interpret this shape. OpenCV loads images in the BGR format. BGR is an abbreviation for three channels Blue, Green, and Read. That is, the image consists of three color channels: Blue (B), Green (G), and Red (R). We can access these color channels and corresponding NumPy arrays by typing
# blue channel
imageOriginal[:,:,0]
# green channel
imageOriginal[:,:,1]
# red channel
imageOriginal[:,:,2]
We can check the max and min number values in the color channels, and the data type by typing
# we can check the max and min values
imageOriginal[:,:,0].max()
imageOriginal[:,:,0].min()
# let us check the data type
imageOriginal[:,:,0].dtype
The maximum value is 255, and the minimum value is 0. The returned data type is “uint8”. That is, the corresponding NumPy arrays are of 8 bit integer type, that is in the range from 0 to (2^8-1).
How to display images in OpenCV and Python
Next, let us learn how to display images in OpenCV and Python. For that purpose, we use the function “cv2.imshow”. The following Python script displays the image on the user screen.
# Let us try to display the original image
cv2.imshow("Original Image", imageOriginal)
# This function will make sure that the
# image is opened until the user presses a key
# and that the Python code is running
cv2.waitKey(0)
# After that, we close all the window
cv2.destroyAllWindows()
To display the image we use the function cv2.imshow. The first input of this function is a string that will be displayed in the bar window. The second input is the name of the image. The call of the function cv2.waitKey(0) will make sure that the display window will be on the user screen until the user presses any keyboard key. If the user presses any of the keys, then the execution of the Python code will be completed. However, the display window showing the image will still be on the user’s screen. With the function cv2.destroyAllWindows() we make sure that the display window is closed after the Python script is executed.
How to resize images in OpenCV and Python
If the image is too large, it will not fit on the screen. Consequently, it is necessary to resize the image such that it fits the screen. There are several methods for resizing the image. To make this webpage tutorial as short as possible, we explain the simplest approach. The Python script given below resizes the image and displays the image on the user screen.
# let us now resize the image, so we can show it on the screen
# there are several approaches
# we can manually set the new width and height
# colNew is the new width
# rowNew is the new height
rowNew, colNew = int(rowOriginal/4), int(colOriginal/4)
imageResized1 = cv2.resize(imageOriginal, (colNew,rowNew))
# check the new shape
imageResized1.shape
# show the resized image
# Let us try to display the original image
cv2.imshow("Resized Image 1", imageResized1)
# This function will make sure that the
# image is opened until the user presses a key
cv2.waitKey(0)
# After that, we close all the window
cv2.destroyAllWindows()
To resize the image, we use
rowNew, colNew = int(rowOriginal/4), int(colOriginal/4)
imageResized1 = cv2.resize(imageOriginal, (colNew,rowNew))
On the basis of the original image size, we define the new size of the image. The width of the image is actually the number of columns of NumPy array representing the image for a single channel. The height of the image is the number of rows. To resize the image, we use the function “cv2.resize”. The first input is the image and the second input is a tuple consisting of the desired dimensions of the resized image. The function “cv2.resize” returns the resized image.
How to save images (PNG or JPG) to files in OpenCV and Python
Finally, let us learn how to save images by using OpenCV and Python. The Python script given below saves the image as JPG and PNG files with different levels of compression and quality.
# save the resized image
# imwrite flag-IMWRITE_JPEG_QUALITY
# goes from 0 to 100 (higher values produce better-quality images)
# max quality
cv2.imwrite("resizedPartsImageMaxQuality.jpg",
imageResized1, [int(cv2.IMWRITE_JPEG_QUALITY), 100])
# medium quality
cv2.imwrite("resizedPartsImageMedQuality.jpg",
imageResized1, [int(cv2.IMWRITE_JPEG_QUALITY), 50])
# min quality
cv2.imwrite("resizedPartsImageMinQuality.jpg",
imageResized1, [int(cv2.IMWRITE_JPEG_QUALITY), 0])
# save the images as PNG files
# imwrite flag-IMWRITE_PNG_COMPRESSION goes from 0 (no compression ) to 9 (max compression)
# max quality
cv2.imwrite("resizedPartsImageMaxQuality.png", imageResized1, [cv2.IMWRITE_PNG_COMPRESSION, 0])
# medium quality
cv2.imwrite("resizedPartsImageMedQuality.png", imageResized1, [cv2.IMWRITE_PNG_COMPRESSION, 4])
# min quality
cv2.imwrite("resizedPartsImageMinQuality.png", imageResized1, [cv2.IMWRITE_PNG_COMPRESSION, 9])
In both cases, to save the image, we use the function cv2.imwrite(). The first input of this function is the name of the file that should store the image. The second input is the name of the image, and the third input is a list specifying the level of compression (in the case of PNG file format) or the image quality (in the case of the JPG file format).
In the case of the JPG file format the quality is defined by using “cv2.IMWRITE_JPEG_QUALITY” flag. The values go from 0 (lowest quality) to 100 (highest quality). The JPG file of the image with the lowest quality is given below.

The image is barely recognizable. However, the advantage of a lower-quality image is that it is stored in a smaller file.
In the case of the PNG file format, the compression level is specified by the flag “cv2.IMWRITE_PNG_COMPRESSION”. The flag cv2.IMWRITE_PNG_COMPRESSION is in the range from 0 (no compression and the highest quality ) to 9 (maximum compression, and the lowest quality). The PNG image with a compression level of 9 is given in the figure below.
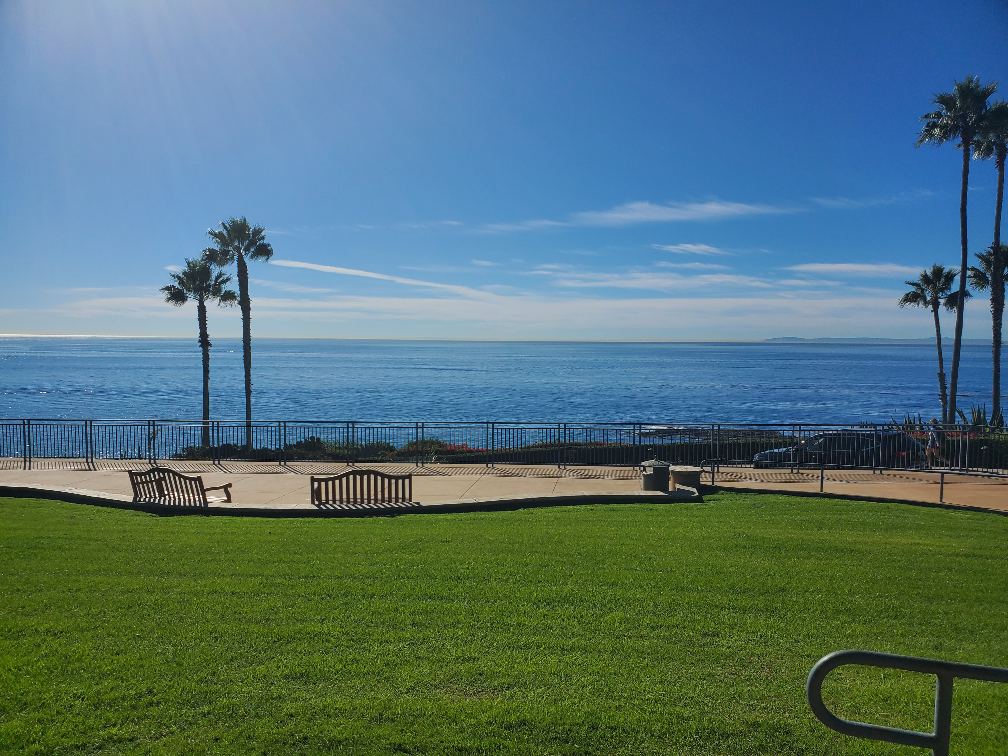