In this Python and OpenCV tutorial, we explain
- How to create a new image with a black background and with desired dimensions in Python and OpenCV.
- How to create a new image with the desired background color and dimensions in Python and OpenCV.
- How to display the created images in Python and OpenCV.
- How to save the created images to image format files, such as PNG or JPG file formats.
The motivation to create this webpage tutorial comes from the fact that we often need to create empty images with desired colors when dealing with computer vision and image processing problems in OpenCV and Python. The YouTube video accompanying this video tutorial is given below.
How to Create a New Image with Black Background in Python and OpenCV
Images in OpenCV are defined by three color channels: Blue (B), Green (G), and Red (R). This format is known as the BGR image format. The order of color channels in this format is different from the more popular Red, Green, and Blue (RGB) channel format. Every channel of an image is defined as a 2D NumPy array consisting of 8-bit integers (uint8), that are in the interval from to
. The complete image is represented by a Numpy array with the dimensions (width, height, color channels).
The Python script below creates a new image with a black background color, displays the image, and saves the image to a PNG file.
import numpy as np
import cv2
# create an empty image
heightImage=600
widthImage=600
# we create a new image by specifying all three BGR channels as zero
# this will create a completely black image
# It should be kept in mind that
# OpenCV loads images in the BGR format
# B - blue, G - green, R- red
newImage = np.zeros((heightImage,widthImage,3), np.uint8)
# display image
cv2.imshow("New Image", newImage)
# This function will make sure that the
# image is opened until the user presses a key
cv2.waitKey(0)
# After that, we close all the window
cv2.destroyAllWindows()
# save image
cv2.imwrite("imageBlack.png",
newImage, [cv2.IMWRITE_PNG_COMPRESSION, 0])
First, we import the necessary libraries: NumPy as np and OpenCV as cv2. Then, we specify the height and width of the image. We create a new image as follows
newImage = np.zeros((heightImage,widthImage,3), np.uint8)
That is, we create a NumPy array with three dimensions of the type “np.uint8”. The NumPy array is filled with zeros. This means that every pixel has color channels set to (0,0,0). This is the BGR code for the black color. We display the image by using
# display image
cv2.imshow("New Image", newImage)
# This function will make sure that the
# image is opened until the user presses a key
cv2.waitKey(0)
# After that, we close all the window
cv2.destroyAllWindows()
The code line “cv2.imshow(“New Image”, newImage)” is used to display the image. The function “cv2.waitKey(0)” is used to complete the script execution when a user presses any key. The function “cv2.destroyAllWindows()” is used to close the image window. To save the image as a PNG file, we use the function
# save image
cv2.imwrite("imageBlack.png",
newImage, [cv2.IMWRITE_PNG_COMPRESSION, 0])
where is the compression level. Generally speaking, the compression level varies from 0 (no compression) to 9 (maximum compression). The generated image is shown in the figure below.

How to Create a New Image with White Background in Python and OpenCV
The Python script given below first creates a new image with a white background in OpenCV. Then, it displays the image.
# let us create an image with a white background
newImageWhite = np.zeros((heightImage,widthImage,3), np.uint8)
# The BGR code for white is (255, 255, 255)
# let us set these channels
# blue channel
newImageWhite[:,:,0]=255
# green channel
newImageWhite[:,:,1]=255
# red channel
newImageWhite[:,:,2]=255
# display image
cv2.imshow("White Image", newImageWhite)
# This function will make sure that the
# image is opened until the user presses a key
cv2.waitKey(0)
# After that, we close all the window
cv2.destroyAllWindows()
To create the image with a white background we need to set all the color channels to 255. We do this by using the following Python script.
# blue channel
newImageWhite[:,:,0]=255
# green channel
newImageWhite[:,:,1]=255
# red channel
newImageWhite[:,:,2]=255
How to Create a New Image with Desired Background Color in Python and OpenCV
Here, we explain how to generate a new image with a desired background color in Python and OpenCV. As an example, we create an image with an orange background. You can easily generalize the presented approach for any other color by finding the BGR color code for that color. First, we create a new image and set the color channels such that they produce the orange background. The BGR color code for the orange color is (0,165,255). Then, we display the image, and finally we save the image. The Python script is given below.
# let us create an image with an orange background
# The BGR code for orange is (0,165,255)
newImageOrange = np.zeros((heightImage,widthImage,3), np.uint8)
# blue channel
newImageOrange[:,:,0]=0
# green channel
newImageOrange[:,:,1]=165
# red channel
newImageOrange[:,:,2]=255
# display image
cv2.imshow("Orange Image", newImageOrange)
# This function will make sure that the
# image is opened until the user presses a key
cv2.waitKey(0)
# After that, we close all the window
cv2.destroyAllWindows()
# save image
cv2.imwrite("imageOrange.png",
newImageOrange, [cv2.IMWRITE_PNG_COMPRESSION, 0])
We independently set every channel of the image such that the resulting background is orange. This is achieved by using the following Python script
# blue channel
newImageOrange[:,:,0]=0
# green channel
newImageOrange[:,:,1]=165
# red channel
newImageOrange[:,:,2]=255
The generated image is shown below.
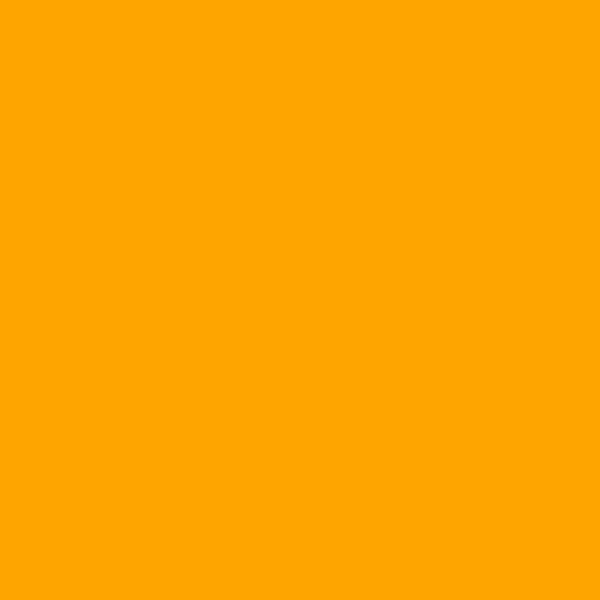
How to Create a New Image with Mixed Background Color in Python and OpenCV
Here, we explain how to create a new image with a mixed background color in Python and OpenCV. The colors are blue (BGR code 255,0,0)) and red (BGR code: (0,0,255)). The Python script is given below.
# let us create an image with one half blue and another half red color
# BGR blue: (255,0,0)
# BGR red: (0,0,255)
newImageMixed = np.zeros((heightImage,widthImage,3), np.uint8)
# blue channel
newImageMixed[0:heightImage//2,:,0]=255
newImageMixed[heightImage//2:heightImage,:,0]=0
# green channel
newImageMixed[0:heightImage//2,:,1]=0
newImageMixed[heightImage//2:heightImage,:,1]=0
# red images
newImageMixed[0:heightImage//2,:,2]=0
newImageMixed[heightImage//2:heightImage,:,2]=255
# display image
cv2.imshow("Mixed Image", newImageMixed)
# This function will make sure that the
# image is opened until the user presses a key
cv2.waitKey(0)
# After that, we close all the window
cv2.destroyAllWindows()
# save image
cv2.imwrite("mixedImage.png",
newImageMixed, [cv2.IMWRITE_PNG_COMPRESSION, 0])
The generated image is given below.
