In this post, and in the accompanying video tutorial, we explain how to convert state-space models into transfer functions. We explain how to perform this conversion analytically and in MATLAB. The YouTube video tutorial accompanying this post is given below.
Consider the following state-space model
(1)
where is the state vector,
is the input vector, and
is the input vector, the matrices
,
, and
are the system matrices.
Our goal is to convert this state-space model to a transfer function (also called a transfer matrix, since the system has multiple inputs and outputs): the transfer function will have the following form
(2)
where is the transfer function and
is the complex variable (obtained by applying the Laplace transform), and
and
are the Laplace transforms of the output and input vectors. Note that the Laplace transforms of vector are denoted by capital letters.
Let us apply the Laplace transform to our original system (1). Since transfer functions are defined for zero initial conditions, we have to keep this fact in account while applying the Laplace transform. As the result, from (1), we obtain
(3)
where is the Laplace transform of the state vector. From the first equation, we have:
(4)
where is the identity matrix. From this equation, we have
(5)
where is an inverse of
. By substituting (5) in the output equation of (3), we obtain
(6)
The last equation can be written compactly
(7)
where is the transfer function
(8)
In the case of single-input-single-output systems, the transfer function is not a matrix, and we can write
(9)
Problem: Find the transfer function of the following system
(10)
Solution:
First, we compute . We have
(11)
The inverse matrix is defined by
(12)
where is the adjugate matrix of
and
is the determinant matrix of
. The adjugate matrix is formed by transposing the cofactor matrix. The cofactor matrix of
is (see the video for the explanation on how to compute the cofactor matrix)
(13)
Consequently, the adjugate matrix is
(14)
The determinant of the matrix is
Finally, our transfer function matrix is:
(15)
Below are the MATLAB codes for computing the transfer function. There are two approaches. Here is the first approach that relies upon the MATLAB Symbolic Toolbox.
% first approach for calculating the transfer function
syms s
A=[0 1 0;
0 0 1;
-1 -2 -3];
B=[10; 0; 0];
C=[1 0 0]
matrix1=s*eye(3)-A
matrix2=inv(matrix1)
transferFunction=C*matrix2*B
The command syms is used to define a symbolic complex variable . The other parts of the code are self-explanatory.
The second approach is given below. This approach relies upon the MATLAB Control System Toolbox.
% second approach for calculating the transfer function
clear, pack, clc
A=[0 1 0;
0 0 1;
-1 -2 -3];
B=[10; 0; 0];
C=[1 0 0];
D=[]
system1=ss(A,B,C,D)
transferFunction=tf(system1)
We define the state-space model by using the MATLAB function ss(). Then we transform such a state-space model into a transfer function form by using the command tf().
Below is the step response of the system computed by using the MATLAB function step().
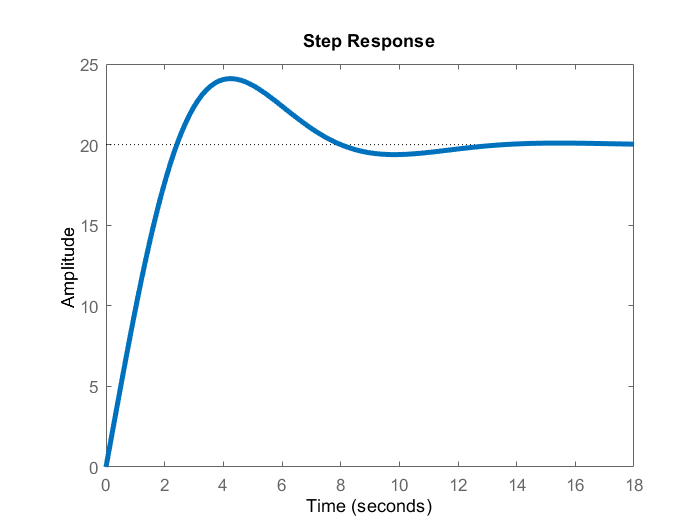