In this Python and Pygame library tutorial, we will learn
- How to draw rectangles in Pygame.
- How to move rectangles around the animation screen in Pygame.
The Pygame library is a powerful library for creating animations and computer games in Python. The Pygame library can also be used for animating the dynamics of mechanical systems, mechanisms, and mobile robots. The YouTube tutorial accompanying this webpage is given below.
Draw a Rectangle is Python and Pygame
Before we explain how to draw a rectangle in Pygame, it is important to understand the coordinate system orientation in the Pygame animation screen. The figure below shows the coordinate system orientation.
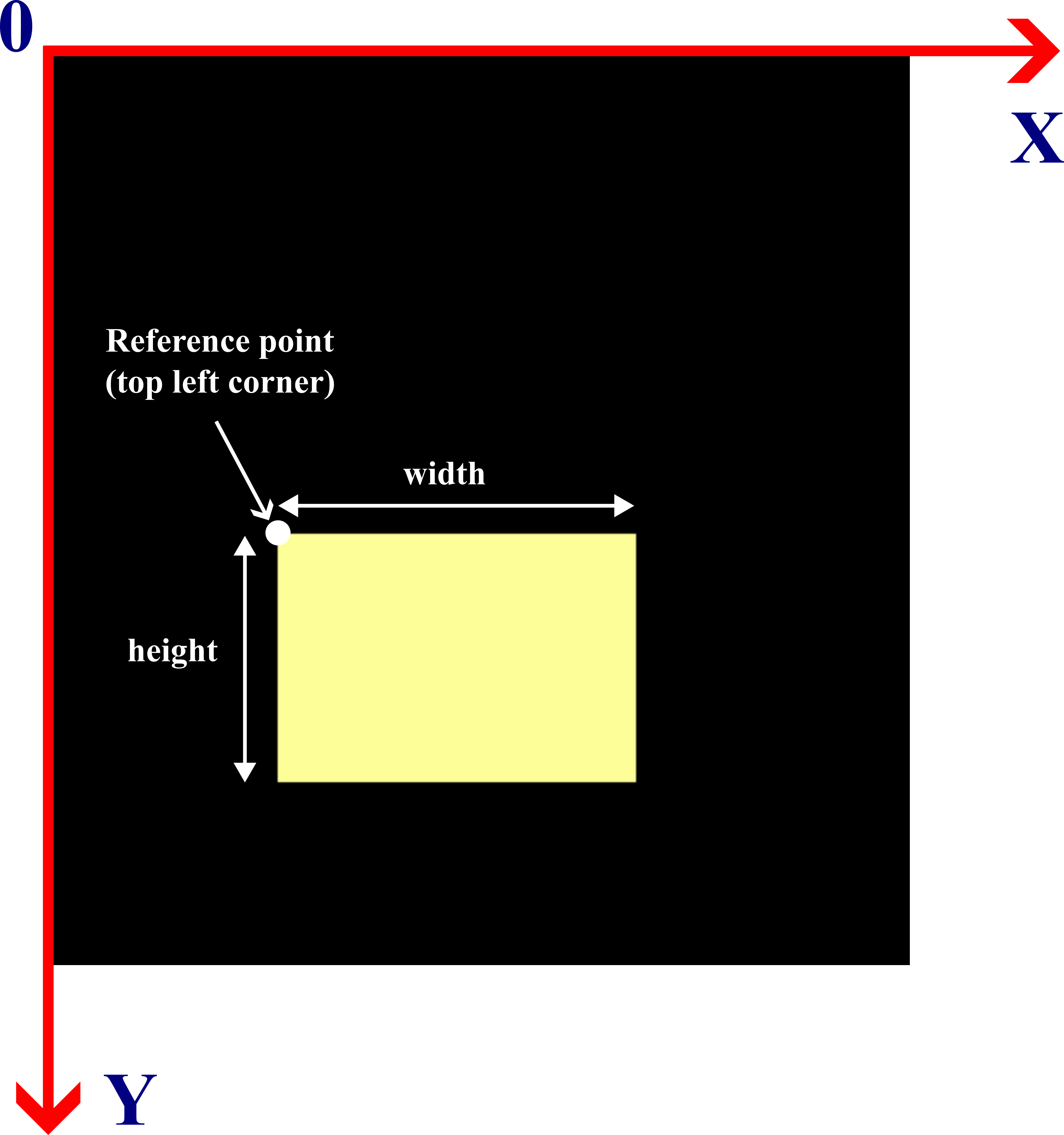
The coordinate axis direction is oriented downward. To define the rectangle in the Pygame library, we need to specify the coordinates of the reference point (top left corner), and the width and height of the rectangle.
The following Python script will create and plot the rectangle.
# Pygame tutorial for drawing a rectangle on the screen
# Author: Aleksandar Haber
# Date: December 2023
# Before running this script make sure that you install Pygame:
# pip install pygame
import pygame
# we import time to display the animation screen for some time
# and them we close the screen
import time
# This function is used to initialize all imported pygame modules.
pygame.init()
# Set window size
size = width,height = 1600, 800
# This function is used to create a display surface
# it returns Surface object to draw on
# Surface is used to represent any image.
screen = pygame.display.set_mode(size)
# create the color tuple for the rectangle
# the color is bright yellow
colorRectangle=(255,255,153)
# over here we draw the rectangle
# x coordinate of the top left corner
# y coordinate of the top left corner
xR=300
yR=400
# rectangle width along the x coordinate
widthR=300
# rectangle height along the x coordinate
heightR=200
pygame.draw.rect(screen,colorRectangle,
(int(xR),int(yR), widthR, heightR))
# This function is used to update Surface image
pygame.display.flip()
# sleep for 20 seconds
time.sleep(20)
# to close the window
pygame.quit()
Here is the explanation of the above-presented script:
- The function “pygame.init()” is used to initialize all imported Pygame modules.
- The code line ” screen = pygame.display.set_mode(size)” is used to create a display surface with the given size. That is, it is used to create “Surface” Pygame object. The variable “size” is a tuple used to define the size in pixels.
- We draw the rectangle by using the function “pygame.draw.rect()”. The first input argument is the reference to Surface object. The second input argument of this function is the color of the rectangle. The third input argument is a tuple. The first two entries of the tuple are
and
coordinates of the upper left reference point of the rectangle (see Fig. 1 above). The third and fourth entries of the tuple are the width and height of the rectangle.
- The function “pygame.display.flip()” is used to generate the image.
- The function “time.sleep()” is used to pause the code.
- The function “pygame.quit()” is used to close the animation window.
Animate Movement of Rectangle
The Python script shown below will move the rectangle along a straight line.
# Pygame tutorial for drawing and moving a rectangle on the screen
# Author: Aleksandar Haber
# Date: December 2023
# Before running this script make sure that you install Pygame:
# pip install pygame
import pygame
# import numpy
import numpy as np
# This function is used to initialize all imported pygame modules.
pygame.init()
# this function creates a clock object that is used to track time
clock=pygame.time.Clock()
# Set window size
size = width,height = 1600, 800
# This function is used to create a display surface
# it returns Surface object to draw on
# Surface is used to represent any image.
screen = pygame.display.set_mode(size)
# create the color tuple for the rectangle
# the color is bright yellow
colorRectangle=(255,255,153)
# cart width and height
rectangleWidth=200
rectangleHeight=100
# x and y coordinates of the top left corner of the rectangle
x=np.linspace(300,800,500)
y=x
# initialize the frame index i
i=0
# simulation while loop
while (i<len(x)):
# Close window event
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = True
# fill the surface with the background color -black
# this is used to erase the previous frame
# if you do not call this rectangles from the previous
# frames will be kept
screen.fill((0, 0, 0))
# draw the rectangle
pygame.draw.rect(screen,colorRectangle,
(int(x[i]),int(y[i]),rectangleWidth,rectangleHeight))
# update the screen
pygame.display.flip()
# introduce a delay
pygame.time.delay(10)
# this is used to limite the runtime
# by using for example
# clock.tick(50) once per frame,
# the script will not run faster than 50 frames per second.
clock.tick(50)
i=i+1
pygame.quit()
The basic idea of this script is to animate the movement of the rectangle frame by frame. In every frame, we create a rectangle at the positions defined by the NumPy arrays and
. The arrays
and
define a straight line. In the sequel, we explain functions that are not explained in the previous section.
- The code line “clock=pygame.time.Clock()” is used to create a Clock object which is used to track time.
- The code lines:
x=np.linspace(300,800,500)
y=x
are used to define theand
coordinates of the line along which the rectangle will move.
- The index of the frame is initialized by the code line “i=0”.
- The while loop iterates through the entries of the array “x”. For every fixed index of the frame, denoted by “i”, we draw a rectangle at the coordinates “x[i]” and “y[i]”. This is achieved by using
pygame.draw.rect(screen,colorRectangle,(int(x[i]),int(y[i]),rectangleWidth,rectangleHeight)) - The function “screen.fill((0, 0, 0))”. This function is used to fill in the screen with the black color at every frame. If that function is not called, then the rectangles from the previous frames will not be erased.
- We use the code line “pygame.time.delay(10)” to introduce the delay.
- We use “clock.tick(50)” to limite the runtime.
- Finally, with “i=i+1” we increment the frame index.