In this introductory field-programmable gate array (FPGA) tutorial, we explain how to turn on LEDs and implement simple digital logic. This tutorial is based on the Digilent Nexys A7-100T FPGA board, Verilog programming language, and Vivado FPGA programming environment. Everything presented in this tutorial can be generalized to other FPGA boards and processors.
In this tutorial, we explain how to implement the digital logic shown in Fig. 1 below. We have two light-emitting diodes (LEDs), denoted by LED1 and LED2, that we want to turn on and off by using three switches, SWITCH1, SWITCH2, and SWITCH3. LED1 is on when both SWITCH1 and SWITCH2 are on. On the other hand, LED2 is on, when SWITCH3 is on. From the boolean logic perspective, we can write:
LED1=SWITCH1 AND SWITCH2
LED2=SWITCH3
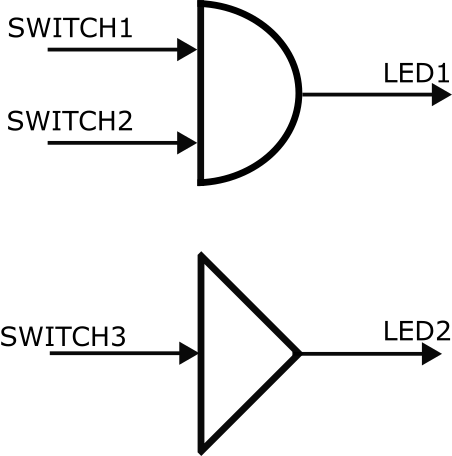
Verilog programming language is a Hardware Description Language (HDL) that is used to model and implement electronic systems, digital systems, and digital circuits. In Verilog, a digital system and logic are implemented as a set of modules. That is, modules are building blocks of Verilog programs. A module corresponding to the logic circuit shown in Fig. 1 is defined as follows
module circuit(input SWITCH1, input SWITCH2, input SWITCH3, output LED1, output LED2);
and(LED1,SWITCH1,SWITCH2); // LED1= SWITCH1 AND SWITCH2
assign LED2=SWITCH3; // LED2=SWITCH3
endmodule
Let us explain this definition. “circuit” is the name of the module. The statement in the parentheses “(input SWITCH1, input SWITCH2, input SWITCH3, output LED1, output LED2)”, represents a port list. The input ports are SWITCH1, SWITCH2, and SWITCH3, whereas the output ports are LED1 and LED2. We use a semicolon symbol (“;”) to terminate the module statement. The module definition should be ended with “endmodule”. Single-line comments can be added by using a double forward slash symbol (“//”). Comments that span several lines can be included by starting the comment section with “/*” and ending the comment section with “*/”.
The statement
and(LED1,SWITCH1,SWITCH2);
defines a logical “and” gate. The arguments of “and” gate, follow a positional notation, where the first argument is the output (in our case LED1), and the other two arguments are inputs (SWITCH1 and SWITCH2). The statement
assign LED2=SWITCH3;
is used to assign the value of SWITCH3 to LED1. In this way, we can ensure that when SWITCH3 is on, LED1 is on.
We have now completely defined the digital logic. However, still have to choose proper switches and LEDs on our FPGA board and define a constraint file.