In this Python and Matplotlib tutorial, we explain how to properly draw circles and how to specify the circle properties, such as face color, edge color, transparency, hatch, center, radius, and other important properties. The YouTube tutorial accompanying this webpage tutorial is given below.
How to Draw Circles in Python and Matplotlib
Geometrical shapes, such as circles, rectangles, polygons, etc., are drawn in Python by using primitive shapes which are known as patches.
To draw a circle in Python, we need to create a circle patch object, and then we need to add the patch to the Axes object. The Python script given below creates a red circle centered at (x=1,y=1)$, and with a radius of 0.5.
import matplotlib.pyplot as plt
plt.figure(figsize=(8,8))
# we draw a circle by specifying the center point, radius, and color
# here we created a patch
# the class is matplotlib.patches.Circle()
circle1=plt.Circle(xy=(1.0,1.0), radius=0.5, color='r' )
# get the current figure
fig = plt.gcf()
# get the current Axes instance
ax = fig.gca()
# add the patch to the Axes
ax.add_patch(circle1)
# set the figure limits
plt.xlim([-2,3])
plt.ylim([-2,3])
# save the figure
plt.savefig('circle1.png',dpi=600)
# show the figure
plt.show()
We create a patch object representing the circle by using the function
circle1=plt.Circle(xy=(1.0,1.0), radius=0.5, color='r' )
The keyword argument “xy” is a tuple defining x and y coordinates of the circle center. The keyword argument “radius” is the radius and “color” is the circle color. After defining the circle patch, we get the current figure and Axes by using “plt.gcf()” and “fig.gca()”. Then, by using the function
ax.add_patch(circle1)
we add the circle patch to the Axes. We then set the x and y axes limits for th plot, save the figure, and show the figure. The figure is given below.
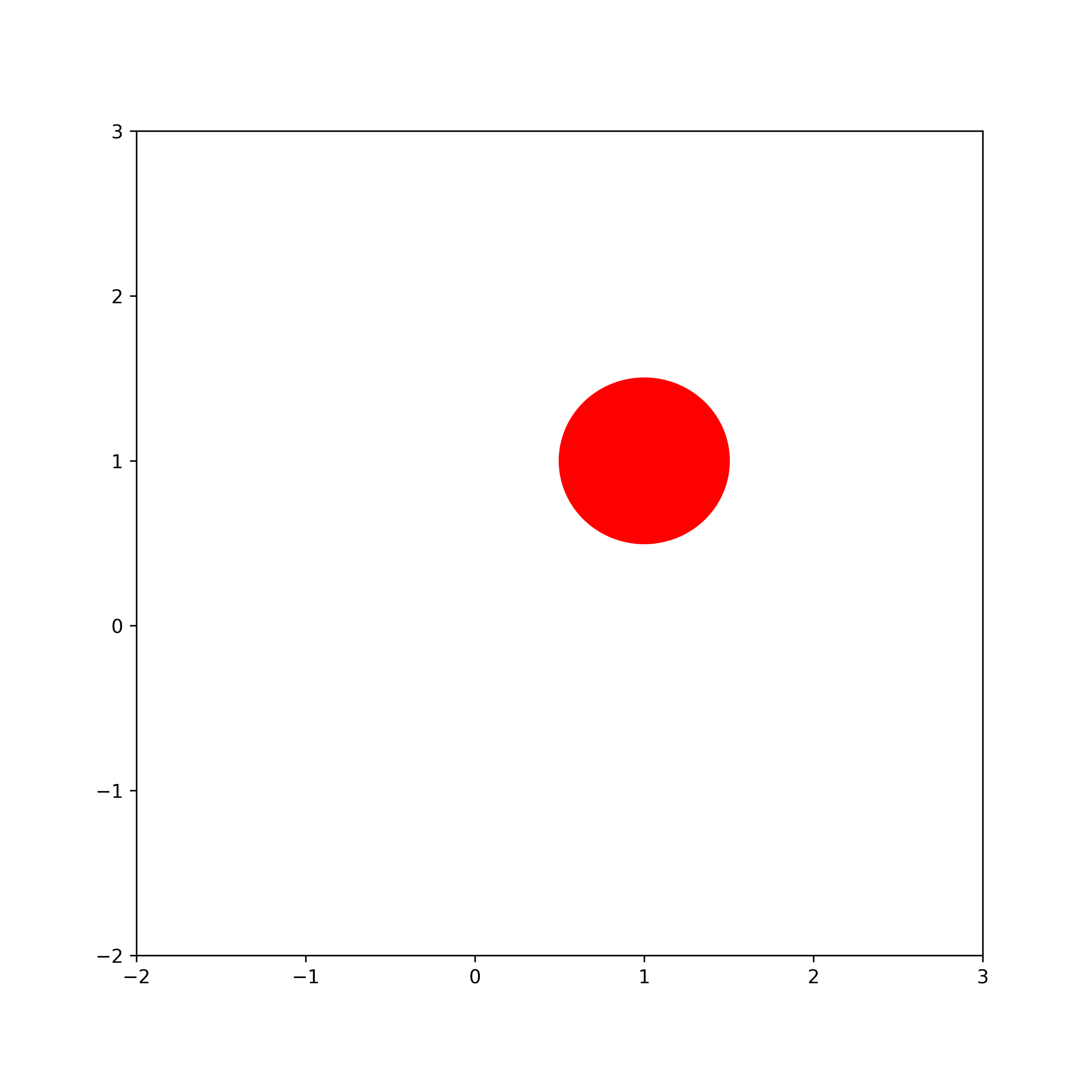
How to Draw a Circle Without a Fill Color (Face Color) and Only With Edge Line in Python and Matplotlb
Next, we explain how to draw a circle without a fill color and only with an edge line in Python and Matplotlib. This script creates a circle only with an edge line
plt.figure(figsize=(8,8))
# we draw a circle by specifying the center point, radius, and color
# here we created a patch
# the class is matplotlib.patches.Circle()
circle1=plt.Circle(xy=(1.0,1.0), radius=0.5, color='r', linewidth=5,fill = False)
# get the current figure
fig = plt.gcf()
# get the current Axes instance
ax = fig.gca()
# add the patch to the Axes
ax.add_patch(circle1)
# set the figure limits
plt.xlim([-2,3])
plt.ylim([-2,3])
# save the figure
plt.savefig('circle2.png',dpi=600)
# show the figure
plt.show()
We achieve this by specifying the keyword argument “fill = False”.
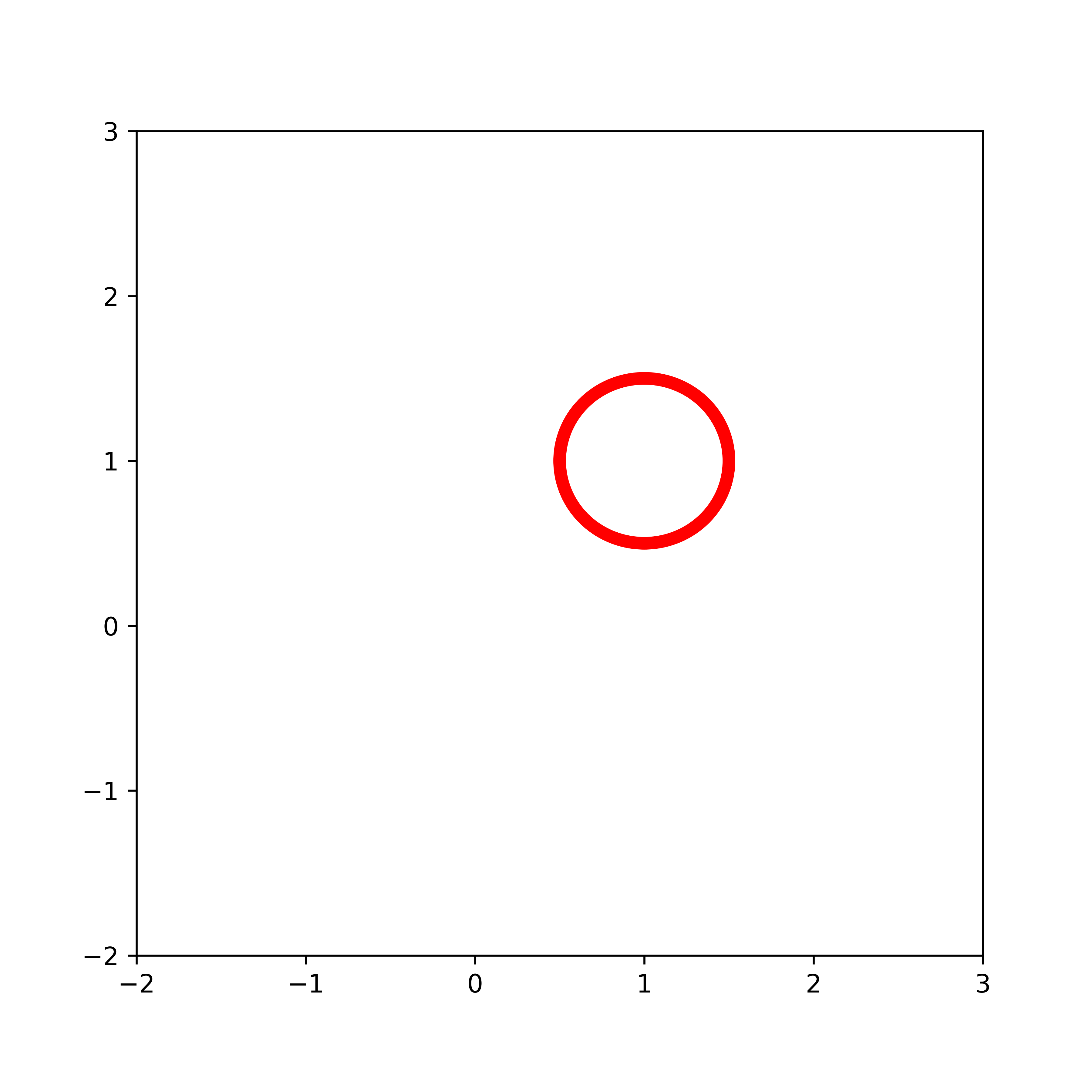
How to Draw Circles with Specific Face and Edge Colors in Python and Matplotlib
The script shown below draws a circle with specified face and edge colors in Python and Matplotlib:
# draw a more fancier circle
plt.figure(figsize=(6,6))
circle1=plt.Circle((1.0,1.0), radius=0.5, alpha=0.6, edgecolor='r', facecolor='b', linewidth=5 )
fig = plt.gcf()
ax = fig.gca()
ax.add_patch(circle1)
plt.xlim([-2,3])
plt.ylim([-2,3])
plt.savefig('circle3.png',dpi=600)
plt.show()
We control the transparency of the circle by using the keyword argument “alpha=0.6”. The figure is shown below.
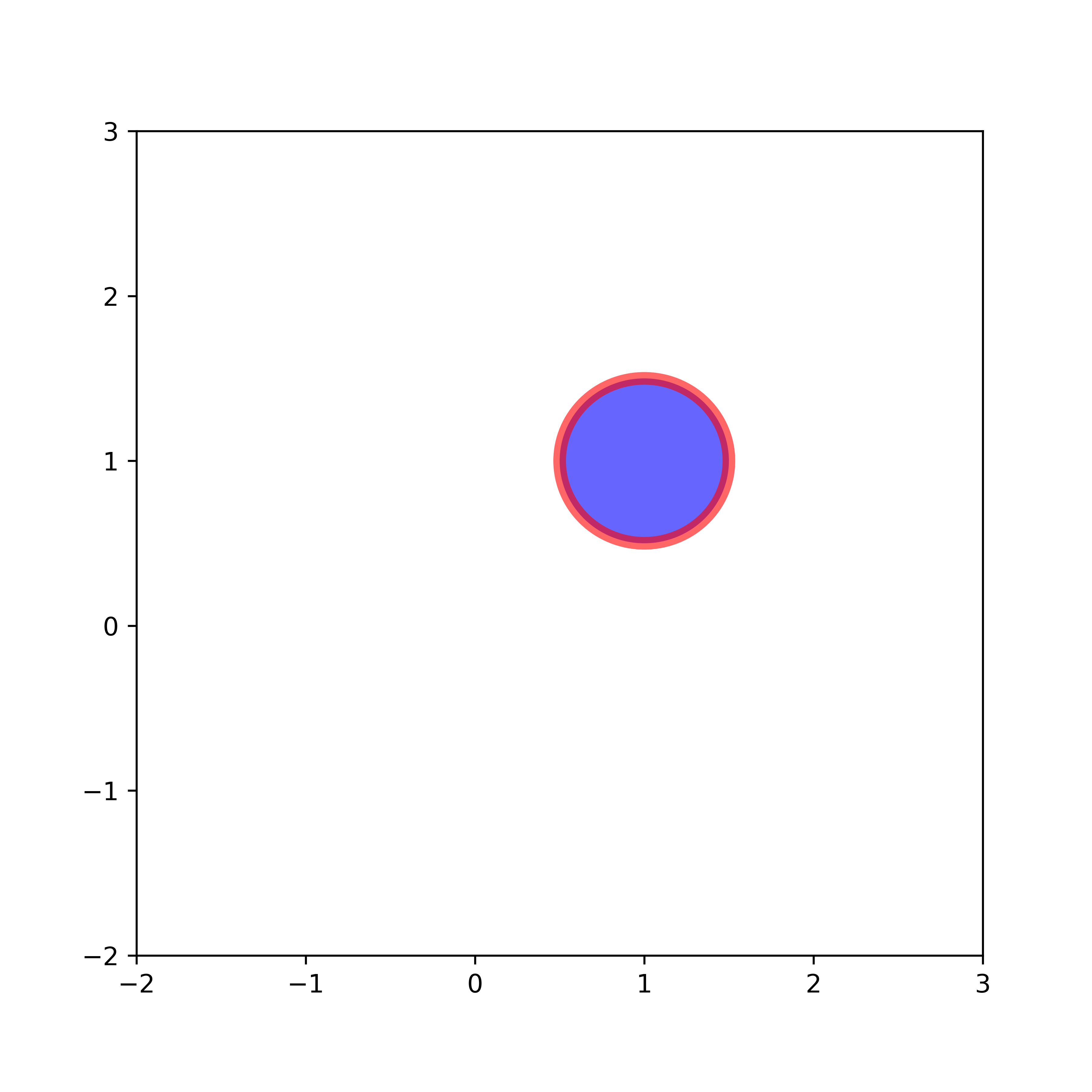
How to Draw Circles With Hatch in Python and Matplotlib
The Python script given below draws a circle with a face hatch in Python and Matplotlib
# draw a circle with a hatch
# hatch can be this
# '/', '\', '|', '-', '+', 'x', 'o', 'O', '.', '*'
plt.figure(figsize=(6,6))
circle1=plt.Circle((1.0,1.0), radius=0.6, alpha=1, facecolor='r', hatch='/', linewidth=5 )
fig = plt.gcf()
ax = fig.gca()
ax.add_patch(circle1)
plt.xlim([-2,3])
plt.ylim([-2,3])
plt.savefig('circle4.png',dpi=600)
plt.show()
We draw a hatch by specifying the keyword argument hatch. The hatch keyword argument accepts the following characters: ‘/’, ‘\’, ‘|’, ‘-‘, ‘+’, ‘x’, ‘o’, ‘O’, ‘.’, ‘*’.
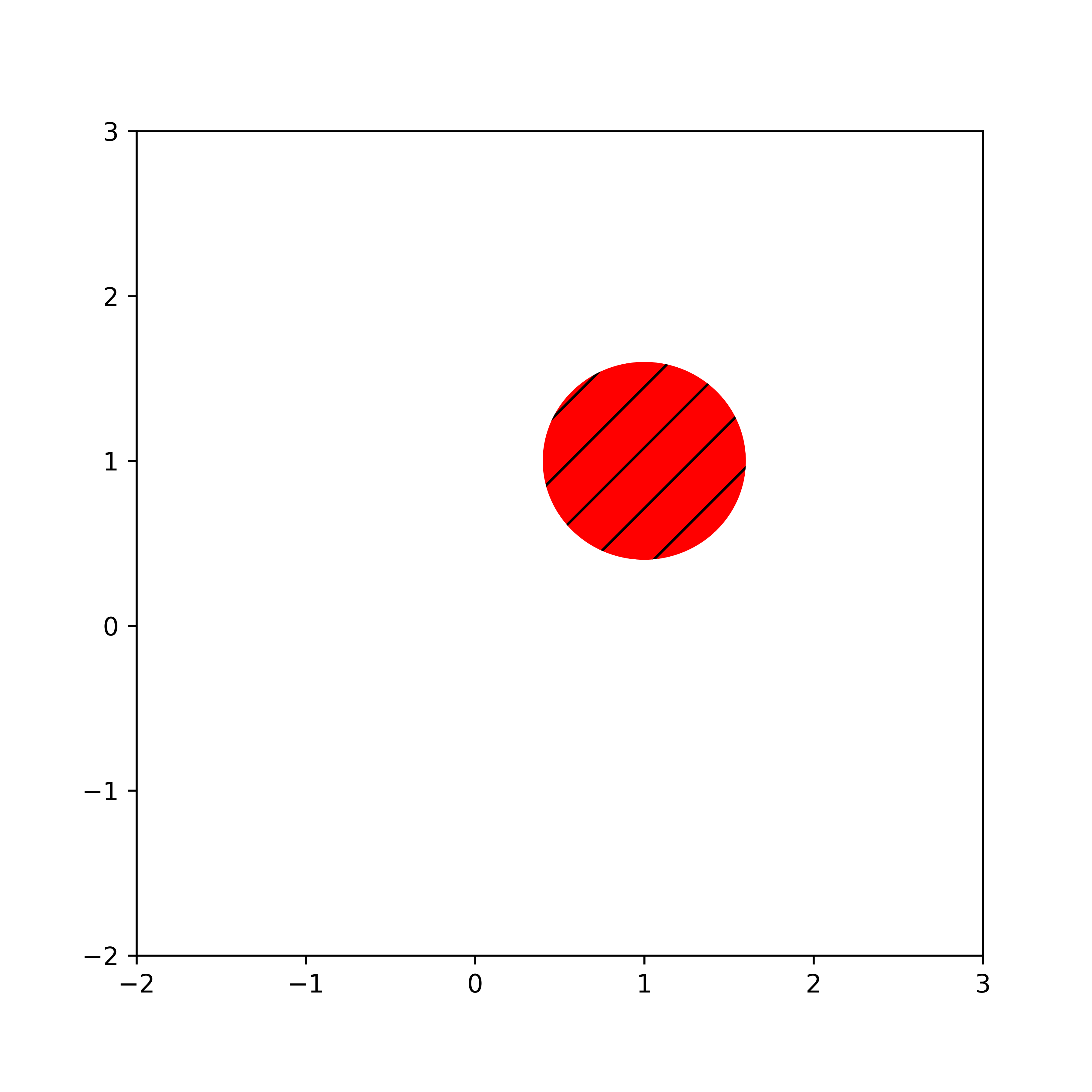