In this post, we explain how to convert thermistor readings into temperatures. We use a least-squares method and we give a MATLAB code. We explain the complete procedure of how to set up a least-squares problem and how to solve it. We also give an example that is based on industrial thermistor. The video accompanying this post, is given below:
In its essence, a thermistor is basically a resistor whose resistance is highly dependent on its temperature. Consequently, we can use them as temperature sensors. Let us assume that we have installed a thermistor and that we have implemented a data acquisition system that is able to give us voltage or current or resistance of the thermistor. For example, if know the voltage and the current, we can compute the resistance using Ohm’s law.
Now, the problem is to convert the computed resistance to temperature. That is we need a calibration curve or a set of points in a resistance-temperature coordinate system that can be used to fit the calibration curve. Usually, a thermistor manufacturer will provide us data for fitting the calibration curve. If we do not have these points, then we can experimentally obtain such points as follows. For example, we can use another temperature sensor (in the sequel we refer to this sensor as a “second sensor”). Assuming that it is accurate, we can place this sensor very close to the thermistor. Then we can obtain a resistance of the thermistor. The temperature measured by the second sensor and the resistance of the thermistor represent a single point on a graph. By repeating this procedure for different values of temperature, we can obtain other points. For example, we can place the second sensor and the thermistor on top of a hot plate whose temperature can be regulated.
To demonstrate how to fit a calibration curve, we will use real-world data. On this website, you can find a set of points (resistance-temperature) for an industrial thermistor ACC101, supplied by Ametherm. This thermistor is of the NTC type, where NTC stands for Negative Temperature Coefficient. That is, for this thermistor type, as the temperature increases, the resistance increases.
Using the data provided on the website, we obtain the following calibration points.
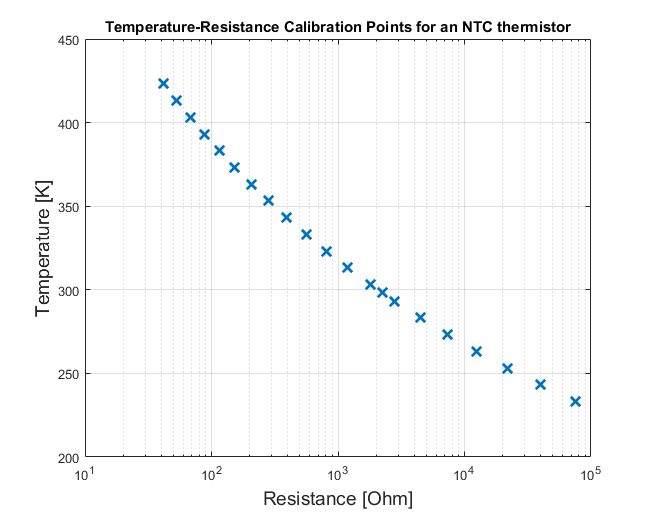
Next, we explain the calibration procedure. In order to fit a function, or better to say in order to fit function parameters that explain the calibration points, we need to have some a priori knowledge. It turns out that the mathematical relation between temperature and resistance for NTC thermistors has extensively been studied. The relation is described by the Steinhart-Hart equation:
(1)
where and
are parameters that need to be estimated,
is the resistance measured in
,
is temperature (absolute temperature) measured in
, and
is a natural logarithm.
The problem of estimating the parameters and
, can be formulated as follows. Given a set of
points
, estimate the constants
and
in (1).
To solve this problem, we use a least-squares method. First, we introduce new variables.
(2)
Consequently, we can transform (1), as follows
(3)
The last equation, can be written in the vector notation
(4)
By substituting

(5)
where


(6)
where



The vector of parameters

(7)
Assuming that


(8)
where
(9)
and where




(10)
The MATLAB code for computing the parameter is given below
clear,pack,clc
% data taken from: https://www.digikey.com/en/products/detail/ametherm/ACC101/5967501?s=N4IgTCBcDaIIYFsCmAXAFkgTggBHAxvgIwAMRO6WCAlgM4oD2mIAugL5A
R = [75780, 39860, 21860, 12460, 7352.8, 4481.5, 2812.8, 2252, 1814.4, 1199.6, 811.40, 560.30, 394.55, 282.63, 206.13, 152.75, 114.92, 87.671, 67.77, 52.983, 41.881];
T =273.15+[-40,-30,-20,-10,0,10,20,25,30,40,50,60,70,80,90,100,110,120,130,140,150];
%T=T(6:end)
%R=R(6:end)
plot(R,T)
% getting the calibration constants:a,b,c
% 1/T=a+b*log(R)+c*(log(R))^3
% y=a+b*x+c*x^3
% x=log(R)
% y=1/T
% Steinhart–Hart equation
%https://en.wikipedia.org/wiki/Thermistor
x=log(R)
z=1./T
s1=numel(R)
for i=1:s1
A(i,1)=1
A(i,2)=x(i)
A(i,3)=x(i)^3
end
parameters=inv(A'*A)*A'*z'
The estimated parameters are ,
, and
. These values are in agreement with the results reported in the Wikipedia page.
Here a few comments are in order. If you know the expected temperature change, then better results are achieved by using the data points that are in the expected temperature range. In the example shown above, we have used a relatively large temperature range that might not produce very accurate results if the temperature range is for example in the range [20,30] Celsius.