In this tutorial, we provide a clear and thorough explanation of dynamic arrays in C++. We first explain the main motivation for introducing dynamic arrays. Then, we explain how to create and delete dynamic arrays. The YouTube tutorial accompanying this webpage is given below.
The need for dynamic arrays in C++
When we declare the classical array in C++:
int a[10];
the array size is fixed when the array is created. That is, the memory space for the array is allocated and fixed at the compile time. The array is allocated on the stack. The memory for variables on the stack is automatically created and deallocated. However, in a number of scenarios, the array size is not known in advance. The solution for this would be to declare very large arrays, such as for example:
int a[1000000];
However, the issue with this approach is that memory will be wasted if we use only a small portion of the allocated memory space for this array.
The solution for this problem is to use dynamic arrays. The dynamic arrays are created during the program execution (at the runtime or execution time). That is, their size and allocated memory space are determined during the program execution. Often, a user is asked for the size of the array, and on the basis of the provided size, the array is created at the program runtime. The dynamic arrays can be created or deleted during the runtime.
How to create and use dynamic arrays in C++
We can define dynamic arrays as arrays which are created during the program execution. They are created by using the operator called “new”. We create them like this
int *p;
p=new int[10];
The statement involving “new” will allocate (reserve)10 memory locations for the array and it will return an address to the first memory location of the created array. That is, it will return the base address of the first element of the array. The memory locations and variables of the array are created next to one another. The memory location returned by “new” is stored in the pointer p. Note that the number 10 over here is fixed, however, we can easily write a program where a user will specify the size of the array at the runtime of the program. The figure below illustrates the creation of dynamic arrays by using the new operator.
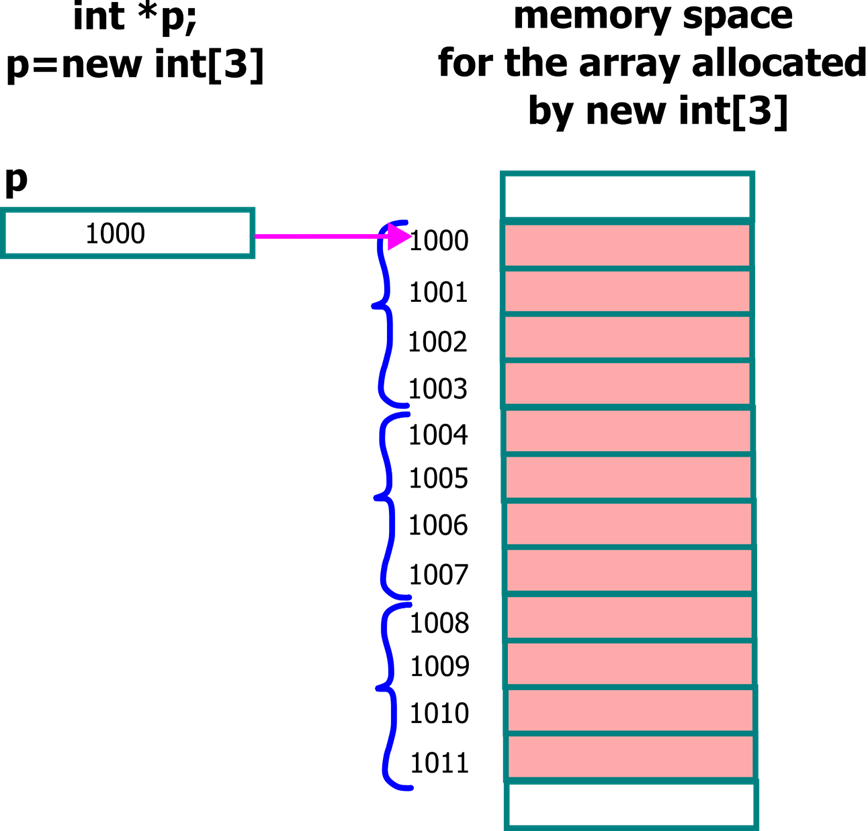
We can use the pointer p to access and change the array elements:
p[0]=1;
p[1]=5;
Or we can use the pointer arithmetic:
*p=1; // this means p[0]=1
p++; // now p points to p[1]
*p=5; // p[1]=5
When using the “new” operator, the arrays are created on the free store. The free store is also called a heap or heap memory.
In contrast to the static arrays whose allocated memory is automatically deallocated after the program is terminated, the memory allocated by using “new” must be deleted by the user. That is, the user has the responsibility to free (erase), the allocated memory. For that purpose, we use the operator called “delete”:
delete [] p;
this statement will free the memory allocated during the statement p=new int[10];
If we forget to free the memory, we might create a memory leak. The memory leak is a serious bug that can create an unstable system.
Suggestion: Whenever you can, use smart pointers instead of “operator new”. More about this in our future tutorials.
C++ Code Demonstrating How to Create Dynamic Arrays
The C++ code given below demonstrates how to create, use, modify, and delete dynamic arrays.
#include<iostream>
using namespace std;
// functon that is used to fill-in the entries of the array
// the user enters the array members, and this function fills them in
void createArray(int array[],int size);
// this function prints the entries of an array
void printArray(int array[],int size);
// this function doubles the stored array values
void changeArray(int array[],int size);
int main()
{
// used to store the total size of array
int arraySize;
// this is the pointer to the first entry of the dynamic array
int *arrayPointer;
cout<<"Enter the size of the array "<<endl;
cin>>arraySize;
// we create a dynamic array of the size given by arraySize
arrayPointer=new int[arraySize];
// read the dynamic array entries from the user input
createArray(arrayPointer,arraySize);
cout<<"Entered array entries are "<<endl;
// print the array members
printArray(arrayPointer,arraySize);
cout<<"I am doubling the array elements "<<endl;
// double the array entries
changeArray(arrayPointer,arraySize);
cout<<"The new elements are "<<endl;
// print the array members
printArray(arrayPointer,arraySize);
// delete
delete [] arrayPointer;
return 0;
}
void createArray(int array[],int size)
{
cout<<"Enter "<<size<<" numbers separated by space and press enter: "<<endl;
for (int index=0;index<size;index++)
{
cin>>array[index];
}
}
void printArray(int array[],int size)
{
for(int index=0;index<size;index++)
{
cout<<array[index]<<" ";
}
cout<<endl;
}
void changeArray(int array[],int size)
{
for(int index=0;index<size;index++)
{
array[index]=2*array[index];
}
}
The code is explained in the comments above the statements.