In this C++ tutorial, we explain how to correctly write numerical values to files in C++. More precisely, we will present a minimal C++ code that will open a file, and then write a numerical data to the opened file. The file will be saved as a text file. Then, to test the file and saved data, we will open a file in Python and read saved data as numpy arrays. Then, we will plot the NumPy array.
C++ Code for Saving Numerical Values From A Data File
The code is given below.
#include<iostream>
#include<fstream>
#include<cmath>
using namespace std;
#define SIZE_ARRAY 1000
int main()
{
double array[SIZE_ARRAY];
ofstream outStream;
outStream.open("data.txt");
float dT=0.01;
if(outStream.fail())
{
cout<<"Could not open the file"<<endl;
exit(1);
}
for (int i=0; i<SIZE_ARRAY; i++)
{
array[i]=sin(3*(i*dT))+cos(8*(i*dT));
cout<<array[i]<<endl;
outStream<<array[i]<<endl;
}
outStream.close();
return 0;
}
This code will store the data in the file called “data.txt”. To save the data, we declare an object of the class called ofstream. ofstream class is used to open files and to save data and text to files. To use this class, we had to include the library fstream. We also included the library cmath since we are using the sin and cos functions in the code. We open the file by calling the method open on the object called outStream. We specify the name of the file as an input parameter of the function. The name of the file is data.txt. If this file does not exist, it will be created. Otherwise, if the file exists, its content will be overwritten.
Then, we check if we were able to successfully open the file. Finally, we use a for loop to fill-in the array, print the data to the screen, and to save the data to the file. To save the data, we use the statement:
outStream<<array[i]<<endl;
This will save the array entries to the file and after that it will start a new line, such that the next entry can be saved in the new line. As the result, we get a file whose content look similarly to this
0.1
0.2
-0.213
-0.4232
...
That is, all the entries are entered row by row. Next, we use the Python code given below to open the data stored in the file “data.txt” and plot the data. The plot is saved in the png file.
# Importing libraries that will be used
import numpy as np
import matplotlib.pyplot as plt
# name of the file
filename = 'data.txt'
# load the data
loadedData = np.loadtxt(filename)
plt.plot(loadedData)
plt.savefig('data.png',dpi=600)
plt.show()
The plotted data is shown below.
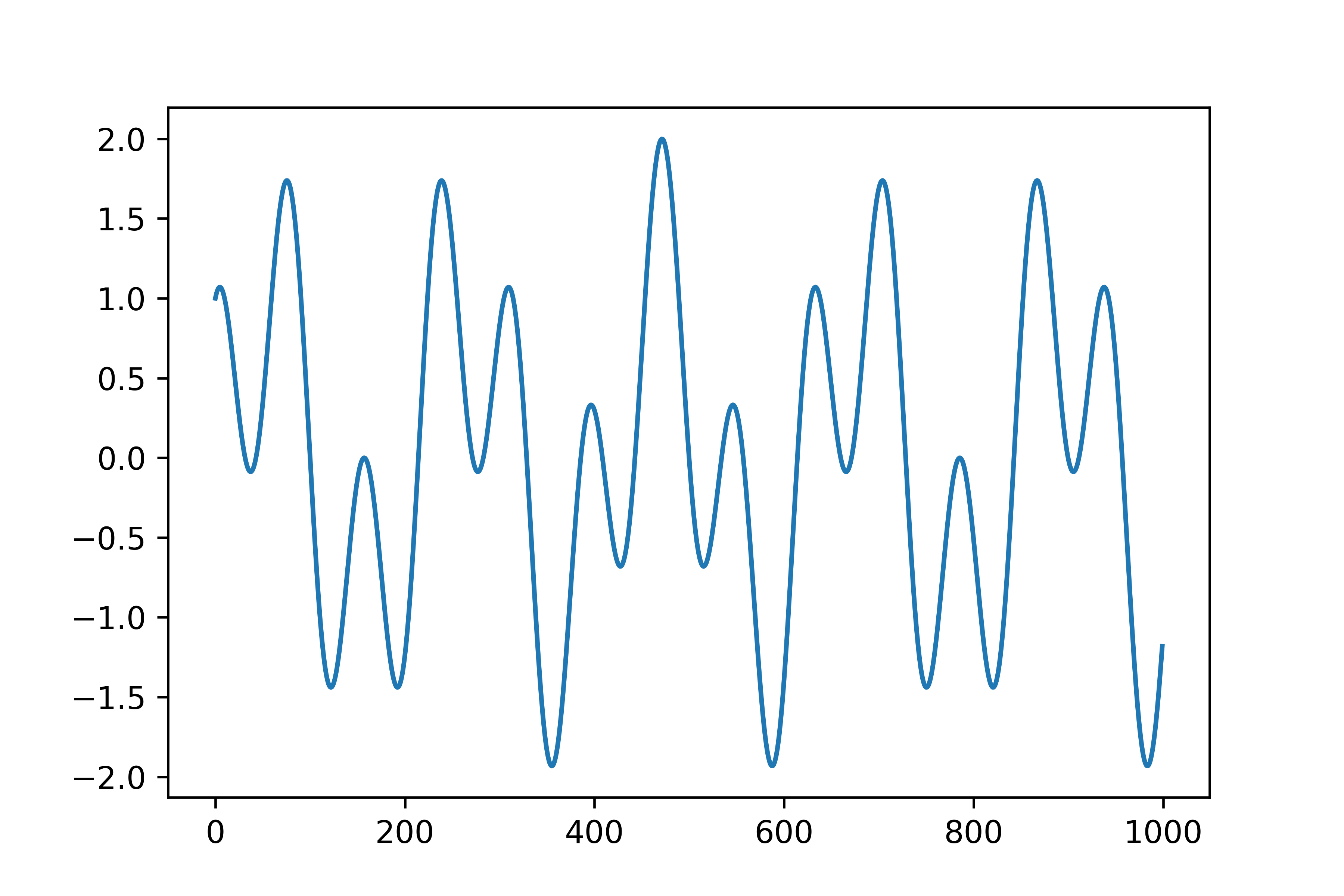