In this C++ tutorial, we explain how to correctly read numerical values from files in C++. More precisely, we will present a minimal C++ code that will open a file containing numerical values and that will sequentially read numerical values from the file. The values that are read will be stored in C++ double data type. The material presented in this tutorial is very important for reading sensor information or for loading numerical data that is saved by some other program. The YouTube tutorial is given below.
C++ Code for Reading Numerical Values From A Data File
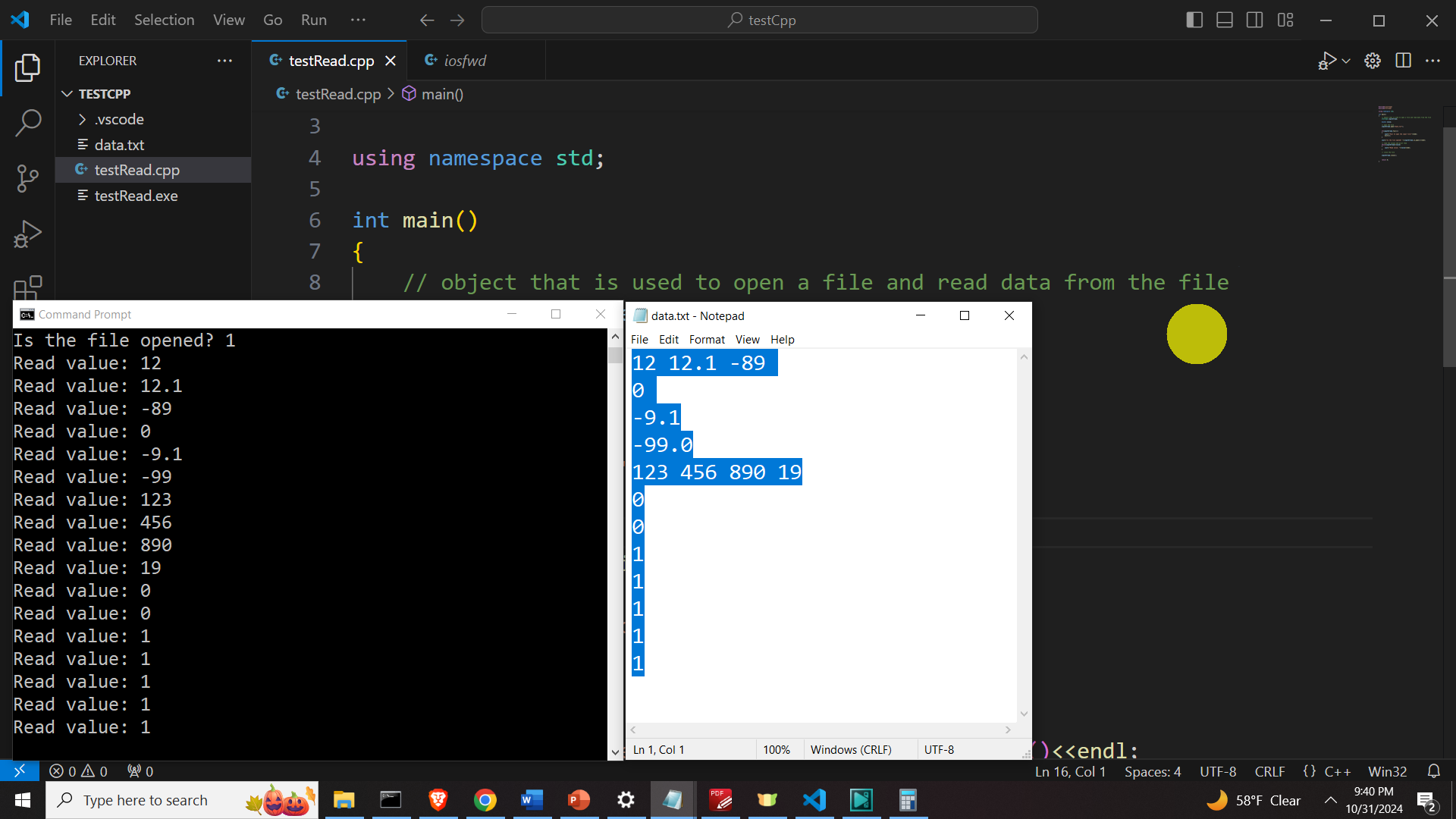
The code file is given below. The code below will open a file called “data.txt”, and it will read double variables from the file and it will display them on the computer screen.
#include<iostream>
#include<fstream>
using namespace std;
int main()
{
// object that is used to open a file and read data from the file
ifstream inputStream;
double value;
// open the file
inputStream.open("data.txt");
if(inputStream.fail())
{
cout<<"Fail to open the input file!"<<endl;
exit(1);
}
cout<<"Is the file opened? "<<inputStream.is_open()<<endl;
// read the values and print them
while(inputStream>>value)
{
cout<<"Read value: "<<value<<endl;
}
// close the file
inputStream.close();
return 0;
}
The data file “data.txt” is given below
12 12.1 -89
0
-9.1
-99.0
123 456 890 19
0
0
1
1
1
1
1
The output is
Is the file opened? 1
Read value: 12
Read value: 12.1
Read value: -89
Read value: 0
Read value: -9.1
Read value: -99
Read value: 123
Read value: 456
Read value: 890
Read value: 19
Read value: 0
Read value: 0
Read value: 1
Read value: 1
Read value: 1
Read value: 1
Read value: 1
Code Explanation
Consider the code block shown below. To open the data file and to read the numerical values from the file, we use declare an ifstream object valled inputStream. Ifstream is a class that is used to open and read data from the files. ifstream (input file stream) class is defined in the library fstream. The variable called value of the double type is used to store the data that is read. Then, we use the created object to open the data file.
// object that is used to open a file and read data from the file
ifstream inputStream;
double value;
// open the file
inputStream.open("data.txt");
Then, we check if the file is opened successfully.
if(inputStream.fail())
{
cout<<"Fail to open the input file!"<<endl;
exit(1);
}
cout<<"Is the file opened? "<<inputStream.is_open()<<endl;
To read the file content and to display it at the output screen, and to close the file, we use the piece of code shown below.
// read the values and print them
while(inputStream>>value)
{
cout<<"Read value: "<<value<<endl;
}
// close the file
inputStream.close();
With the statement
inputStream>>value
We read the numerical value and store it in the file. Note that we are using the extraction operator >> (extract the operator) to read the numerical value. If the value is successfully read, the above statement returns 1, otherwise it returns 0. Zero is returned if the end of file is reached. By putting the last statement in a while loop, we are able to read the file until the end of the file. After we read the content and print it on the screen, we close the file.