In this tutorial we explain
1) How to install VS Code and Python on a Windows computer.
2) How to write and execute Python code by using VS code and Python virtual environments. That is, we explain how to correctly run Python code inside of a virtual environment.
Important note: whenever you can, write and execute Python code inside of a Python virtual environment. This is a suggested approach for creating disciplined and clean Python projects.
The YouTube tutorial is given below.
The first step is to install Python. Go to the official Python website and download the installation file.
https://www.python.org/downloads
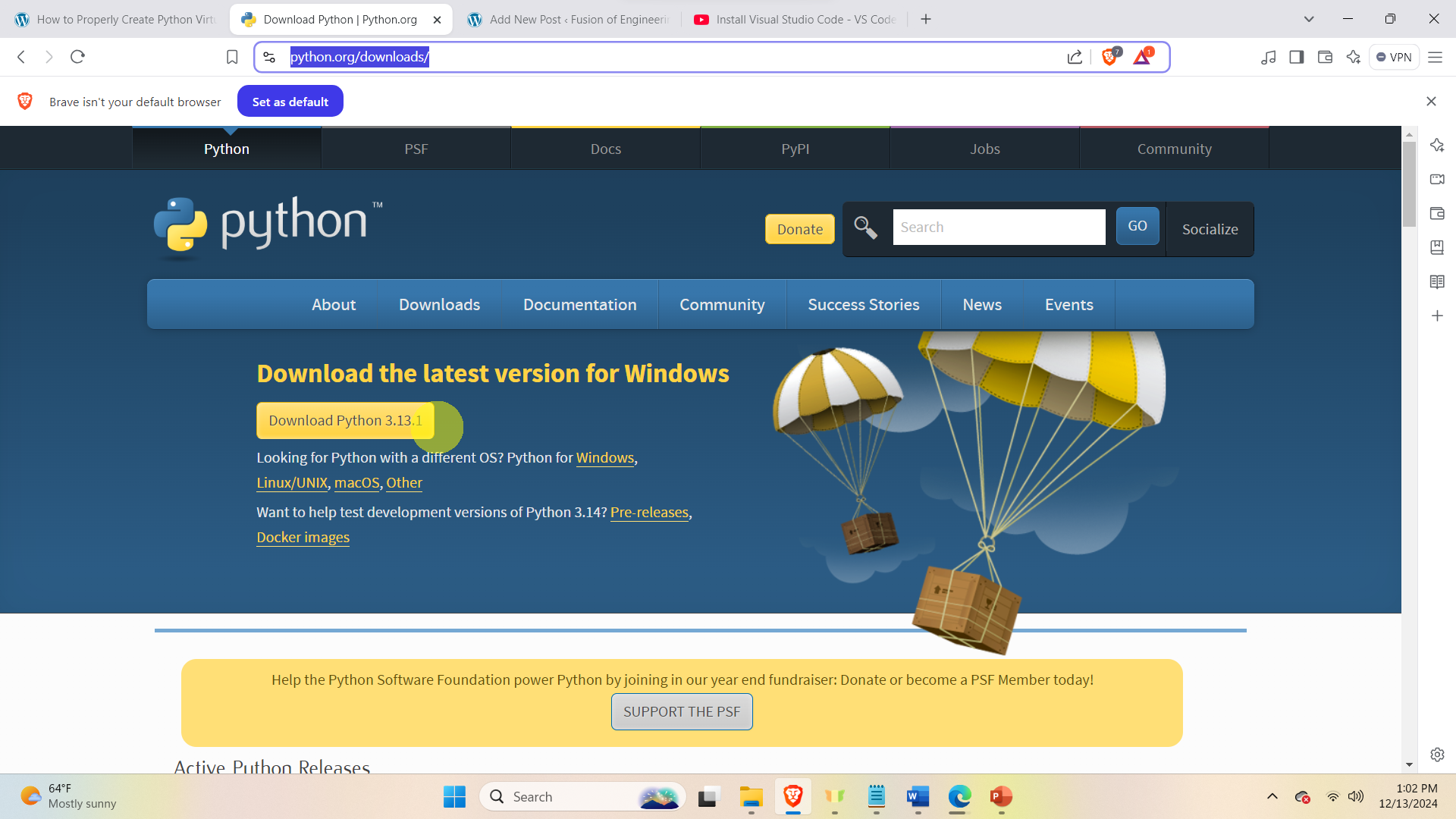
Run the installation file. Make sure that the Python is added to the Windows path. To make sure that Python is installed, open the Command Prompt and type:
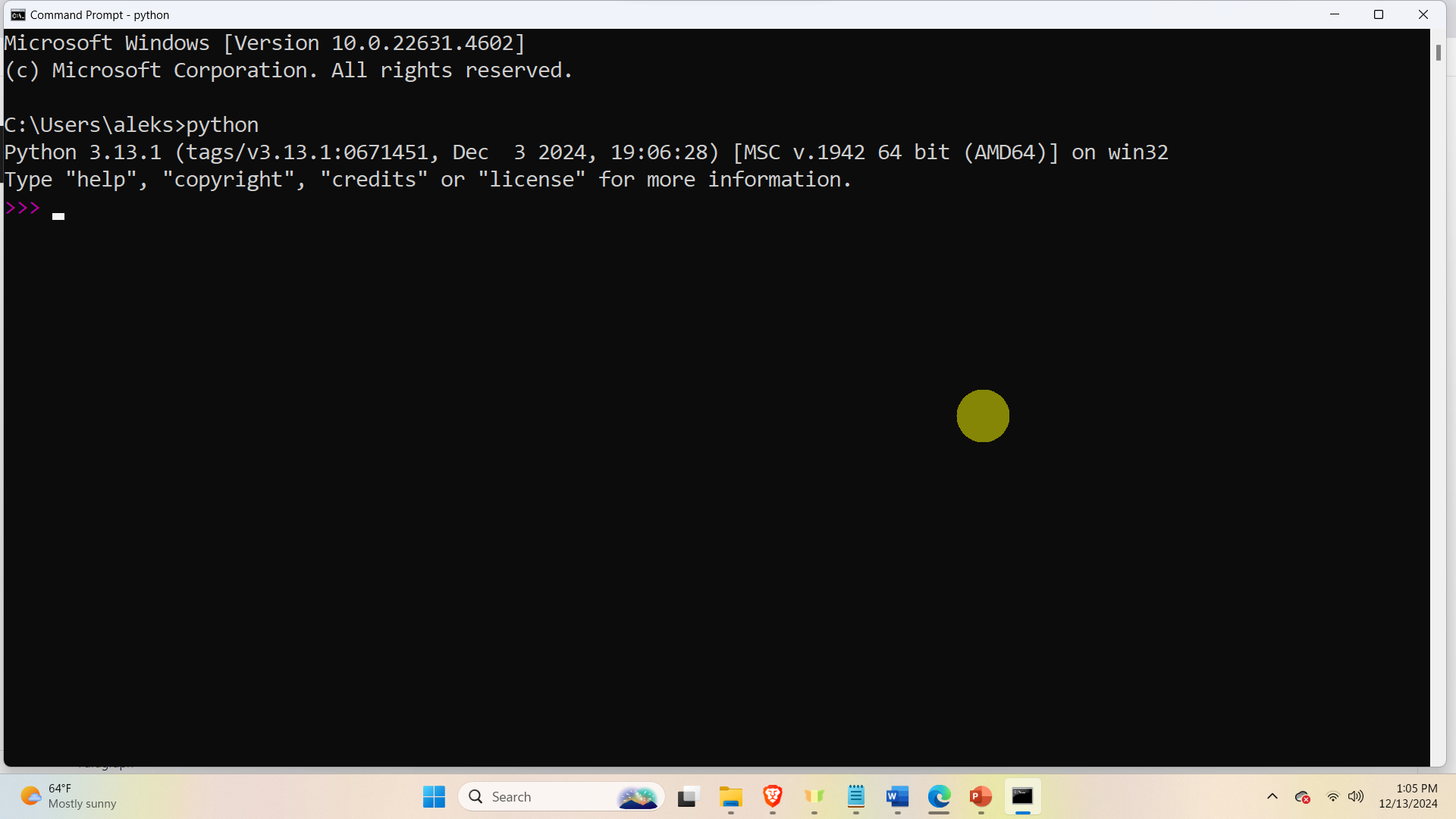
If Python is installed, the Python command line will start and you can enter Python commands. Next type exit to exit Python and close the Command Prompt.
The next step is to download and install Visual Studio Code (VS Code). To download the VS Code go to the official website
and click on Download
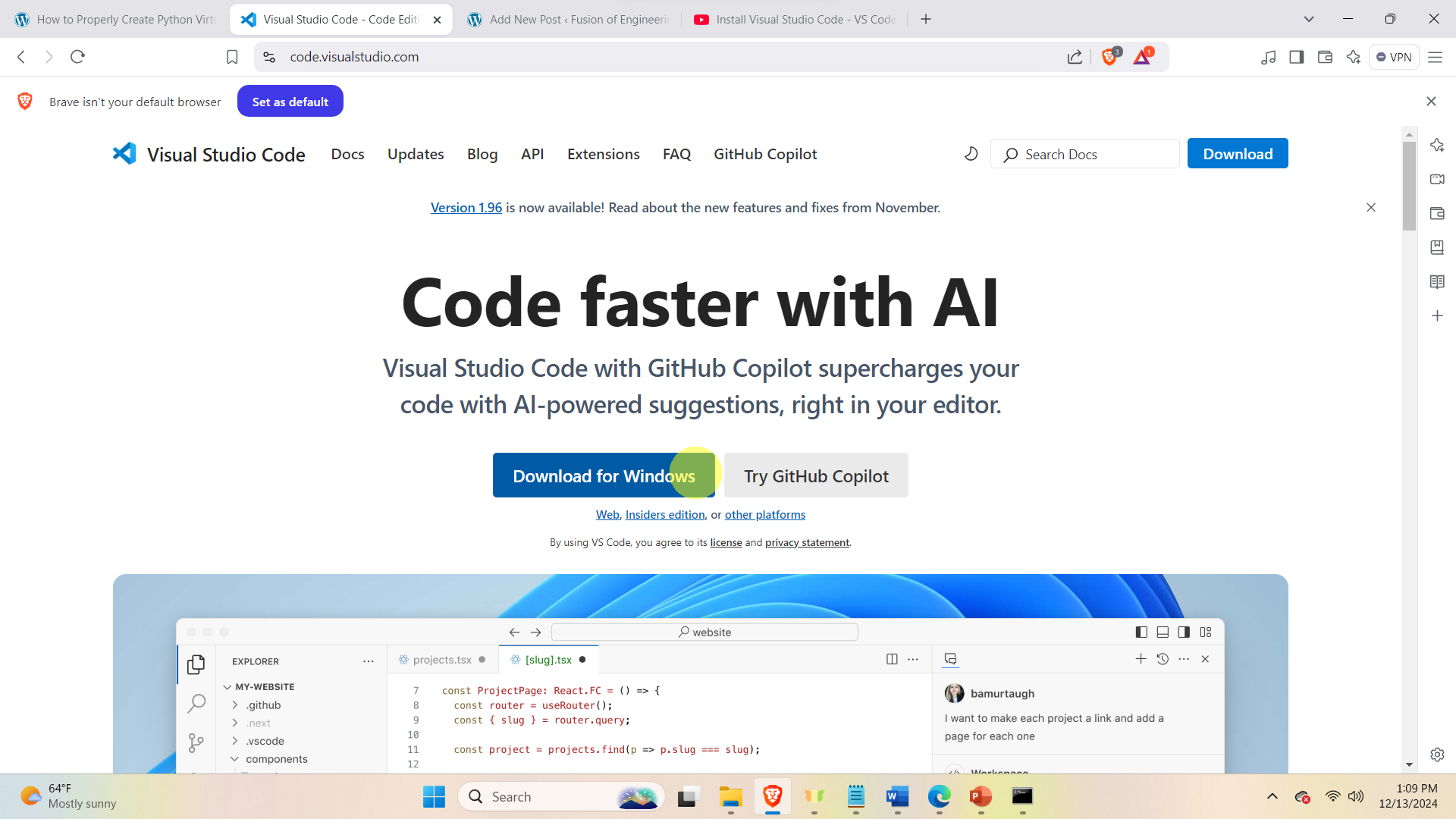
and install VS Code.
Once VS code is installed, open it from the start menu, and install the Python extension for VS Code. To do that first click on File, then Preferences, and then Extensions.
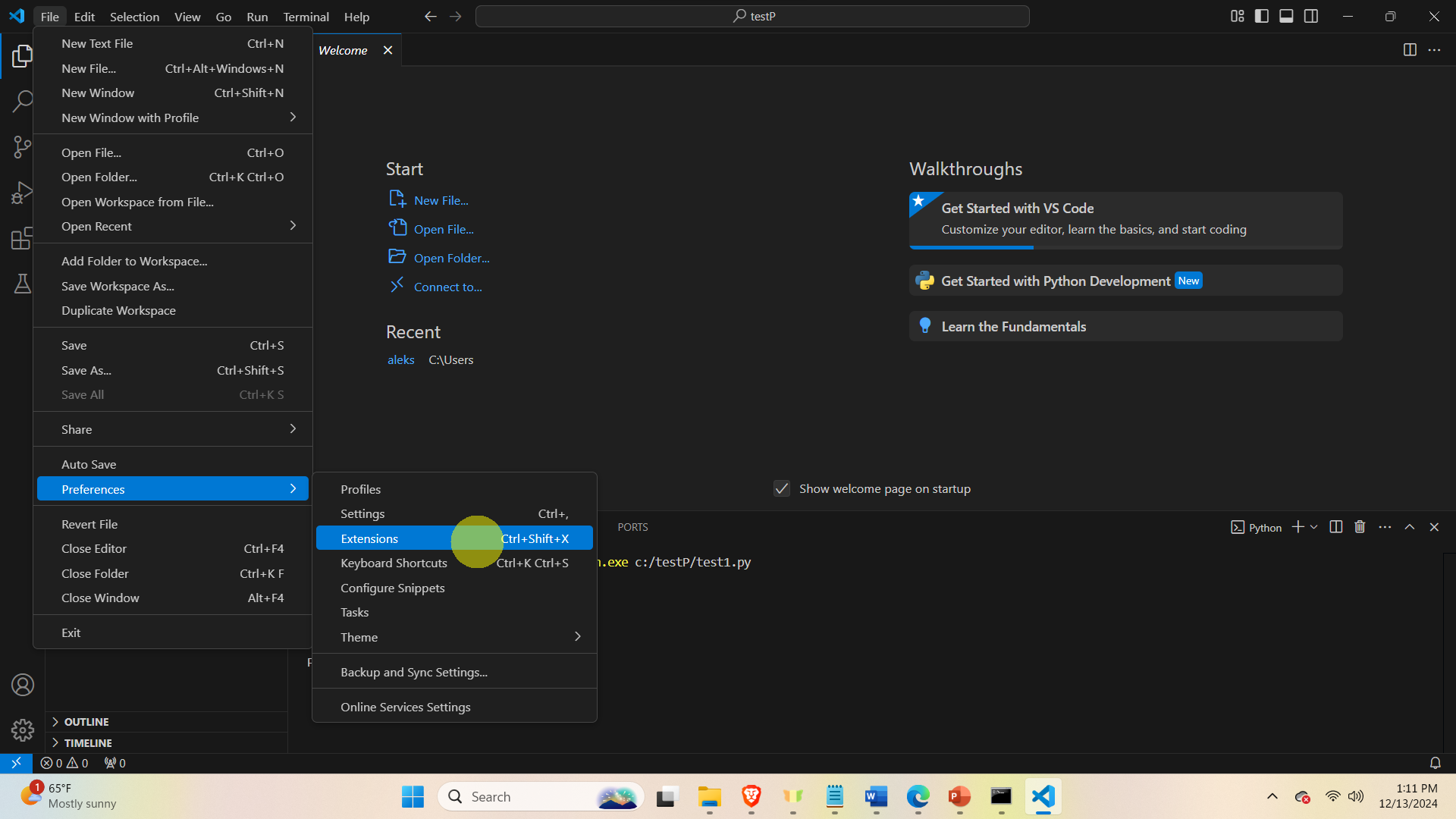
After that search for Python extension, and install it
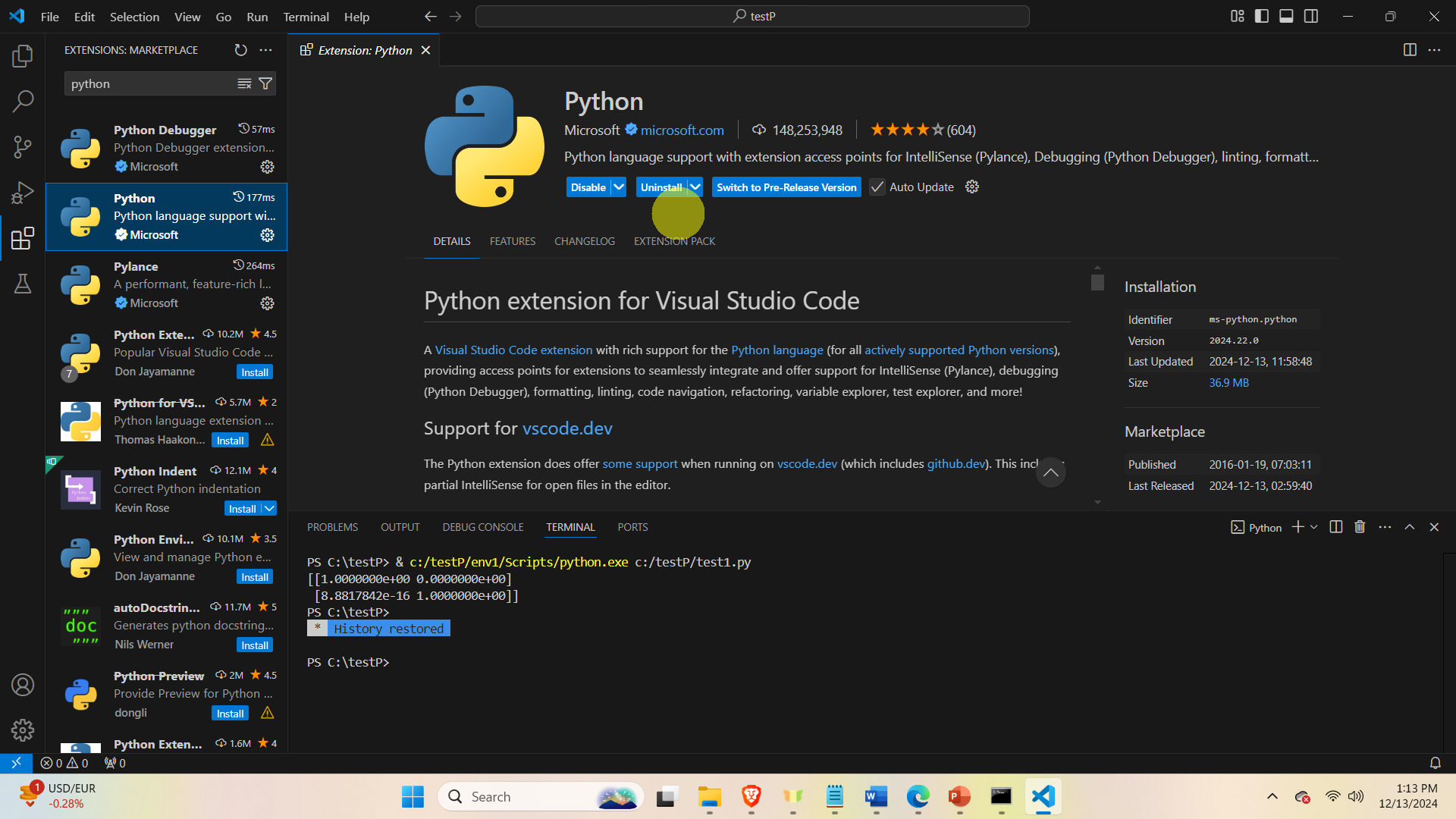
Next, close VS code. Then, open Command Prompt, and type
cd\
mkdir codes
cd codes
python -m venv env1
env1\Scripts\activate.bat
pip install numpy
code .
These commands create a folder called “codes” and create a Python virtual environment inside of that folder. Then, the virtual environment is activated, and the NumPy library is installed. Finally, VS Code will be started.
Then, inside VS Code create a new Python file by clicking on File -> New File, and give the name to the new Python file. Then, type the following Python code:
import numpy as np
A=np.array([[1,2],[3,4]])
B=np.linalg.inv(A)
C=np.matmul(A,B)
print(C)
This code will import the NumPy library, define matrix A and its inverse, and confirm that NumPy can correctly invert matrices. To execute this file inside of the VS Code environment, you first need to press and hold CTRL+SHIFT+P, and then search for Python: Select Interpreter, and after that select the Python interpreter inside of the virtual environment (look at the following two print screens)
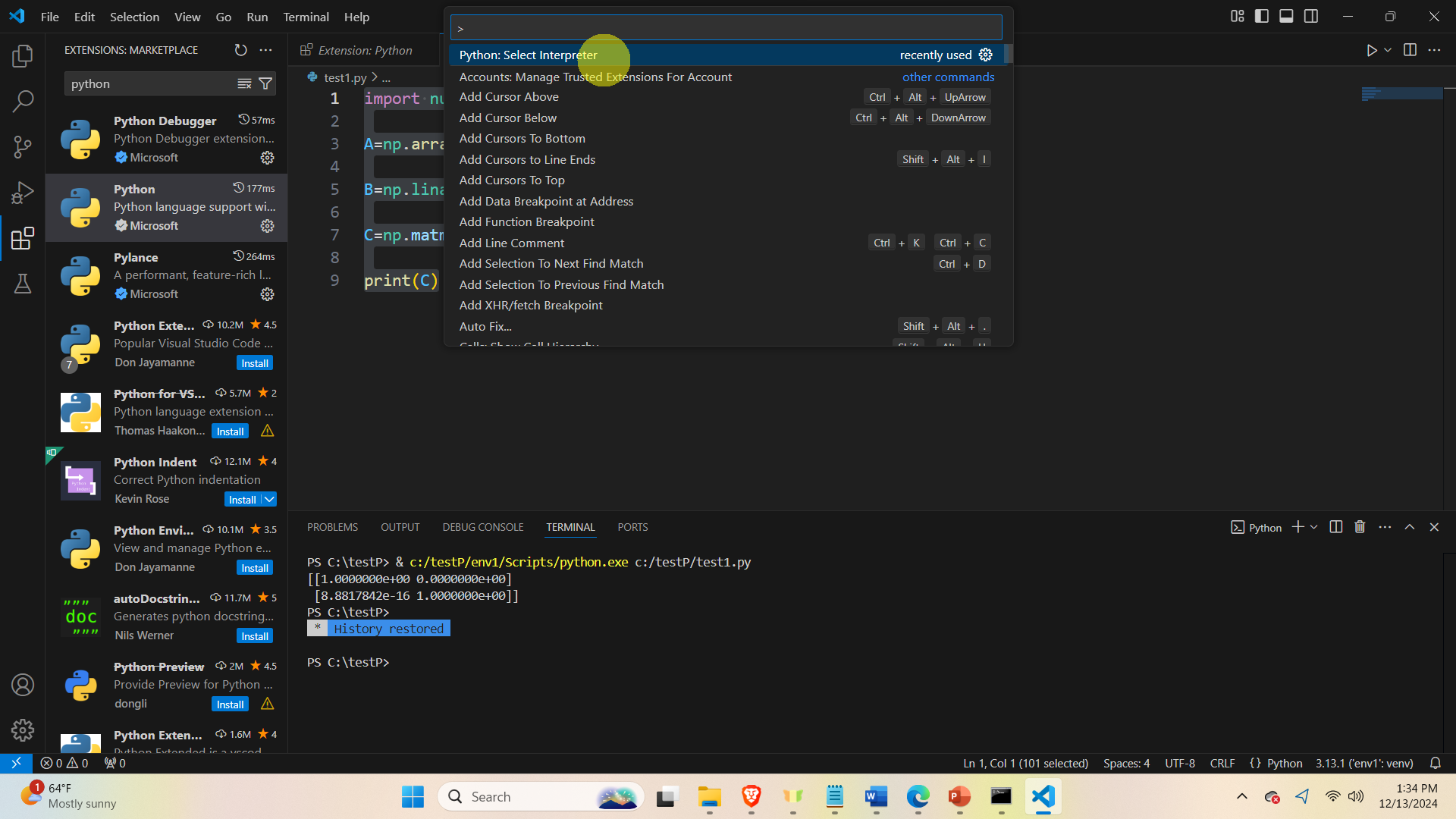
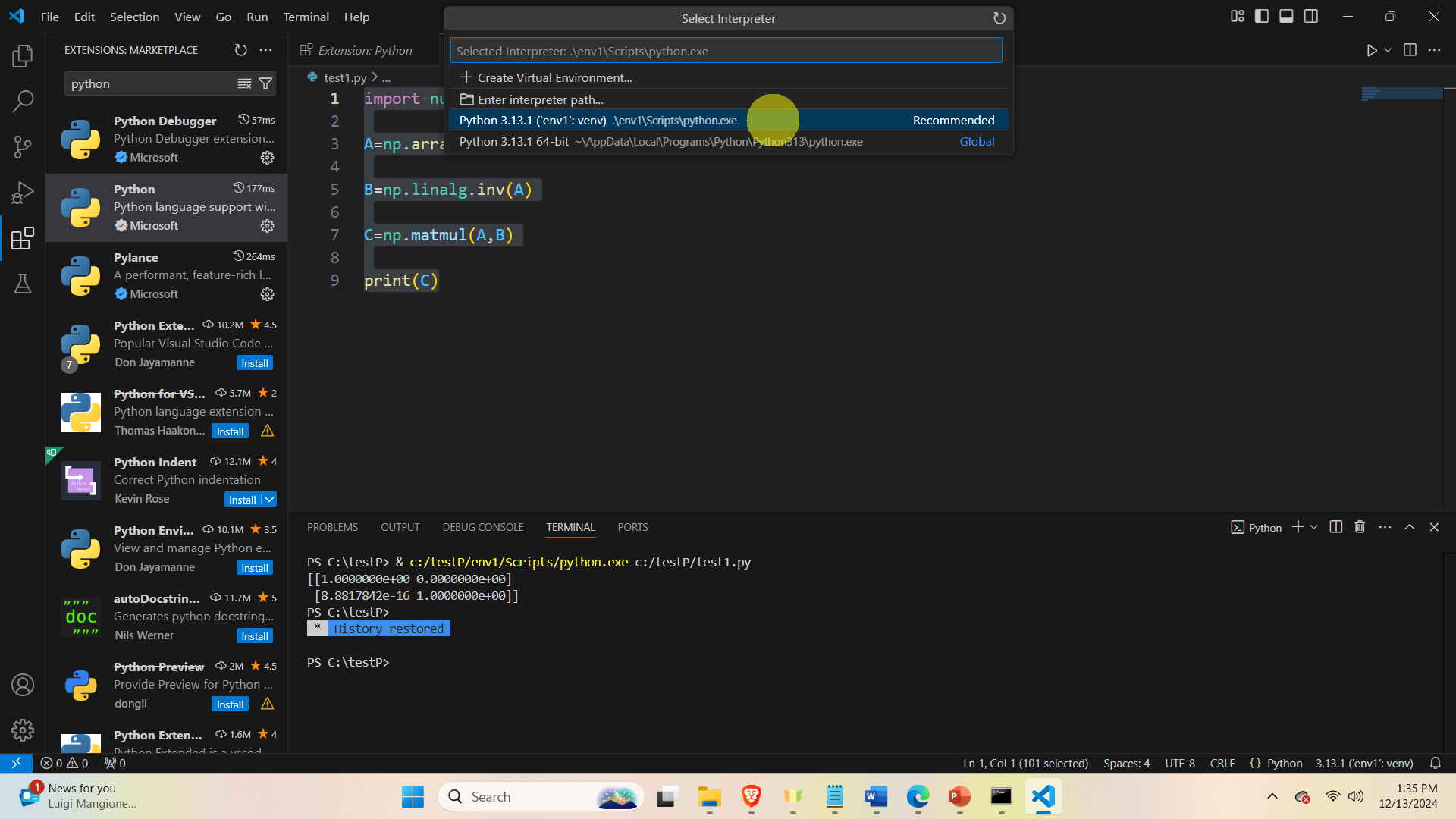
After that you can run the Python code by clicking on the triangle in the top right part of the screen and clicking on Run Python file (see below)
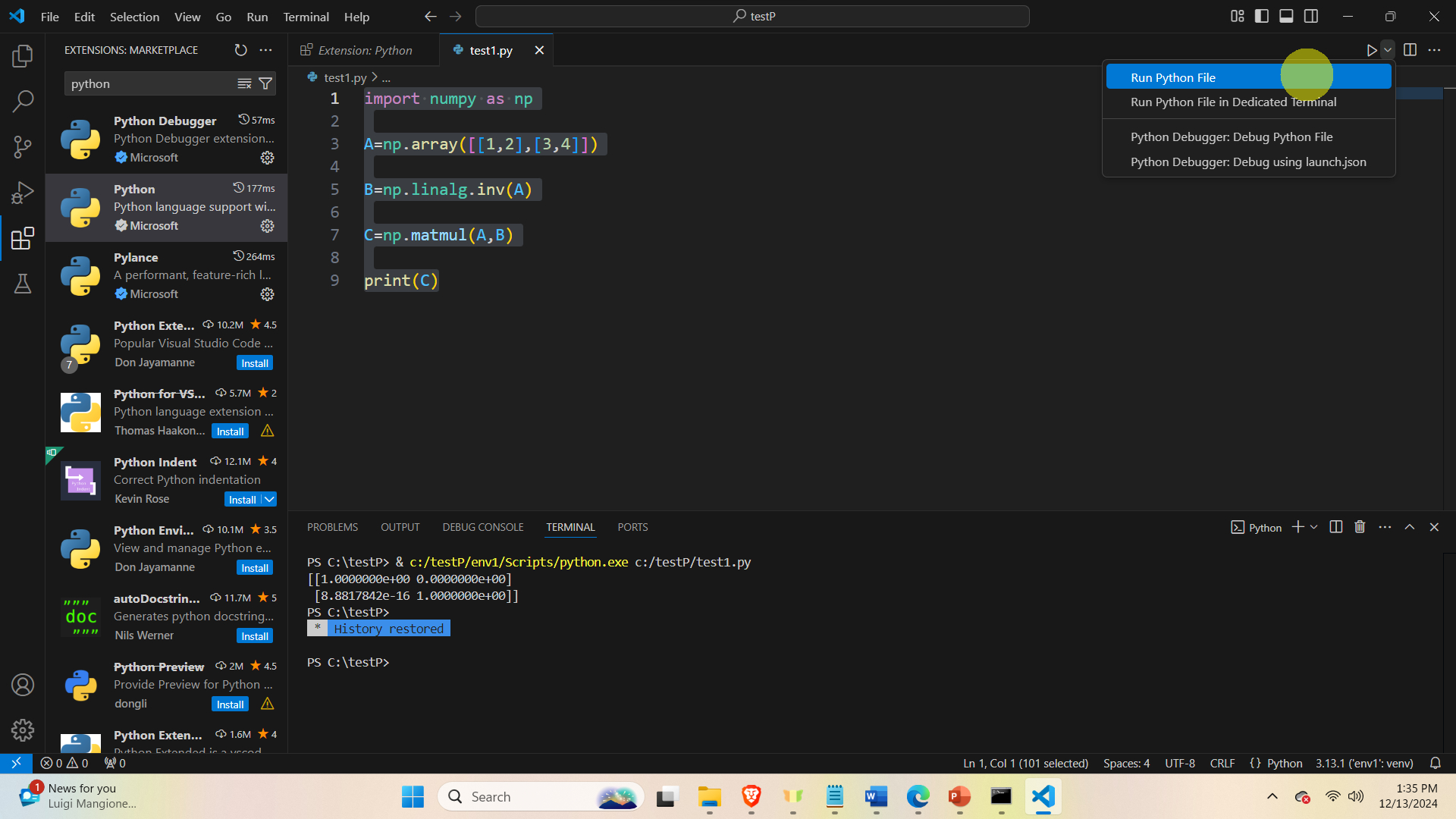
The Python code will be executed
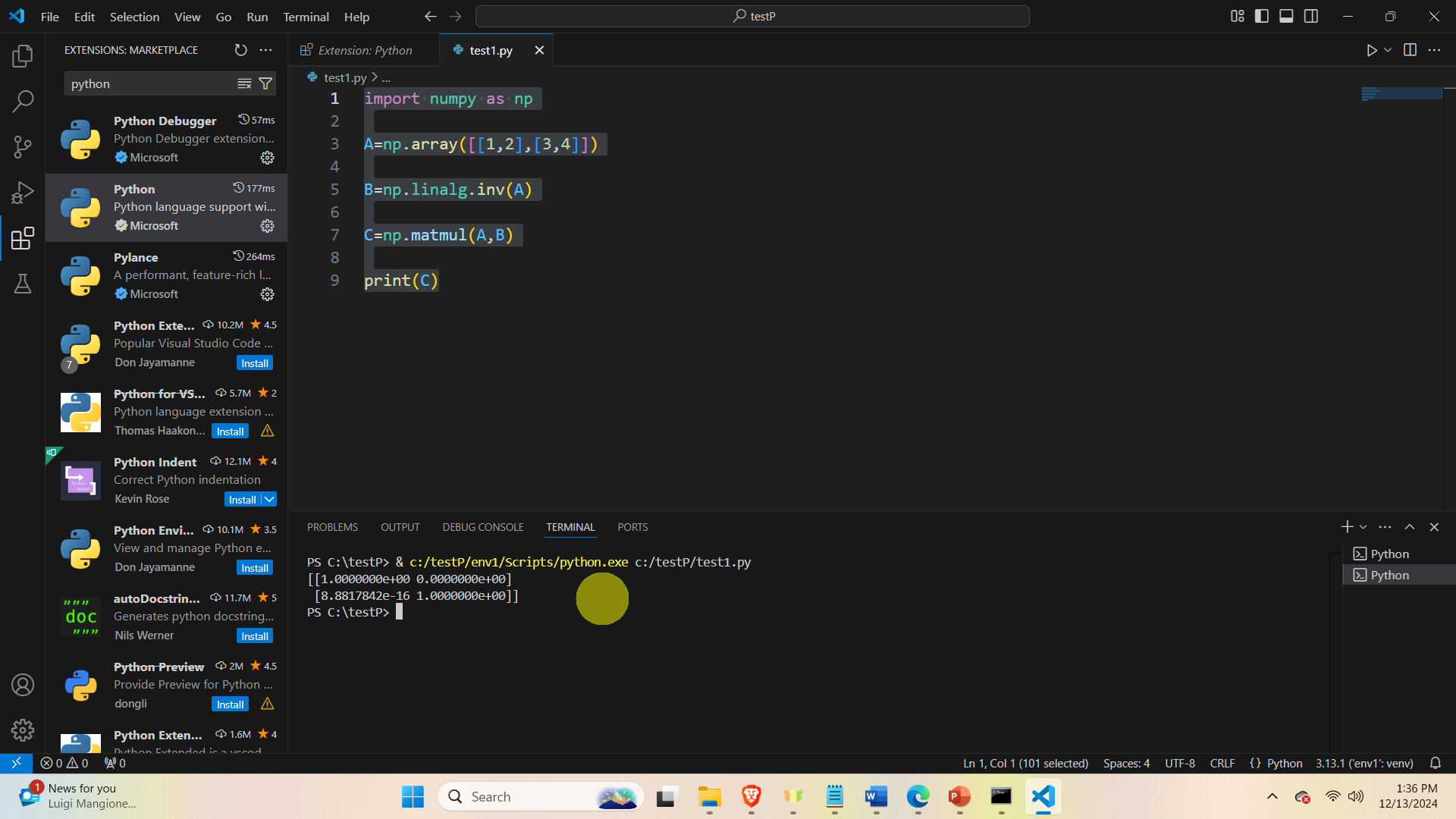